Template for List Page

The HTML template for the list page is used to display a list of items processed by ListView. There are three essential parts that you need to understand to make a template for ListView.
1. object_list
object_list
is a predefined attribute by Django. It is used to bridge between ListView and a template (e.g., list.html).
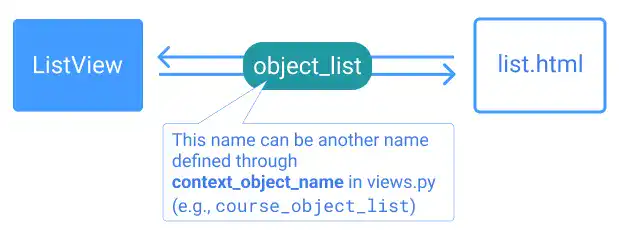
You can use another name for object_list
to make it more intuitive. When you design the view, the additional name can be set through the context_object_name
attribute. In the case of CourseList that we defined earlier (Generic View Basic Attributes), course_object_list
is the name for the list data provided by the LearningCourse model.
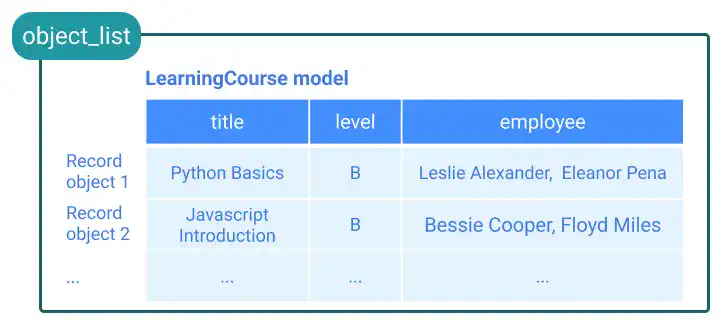
2. {% for ... in object_list %} ... {% endfor %}
As explained on the previous page, you can add simple logic in Django templates using the Django Template Language. When we make a template for ListView, we usually use the for
statement.
In the main figure example, the course
is a variable for the for statement. It can be any string. In this case, the part sandwiched between {% for ... in object_list %}
and {% endfor %}
is iterated.
3. Object attribute to be displayed
The {{ course.name }}
part defines the actual data to be displayed. The 'course
' is a variable defined in the for
statement. Due to the for
statement, each record object in the table is processed one by one. The .title
part is an attribute of 'course
' that specifies which data field (or data column) of the table is displayed.
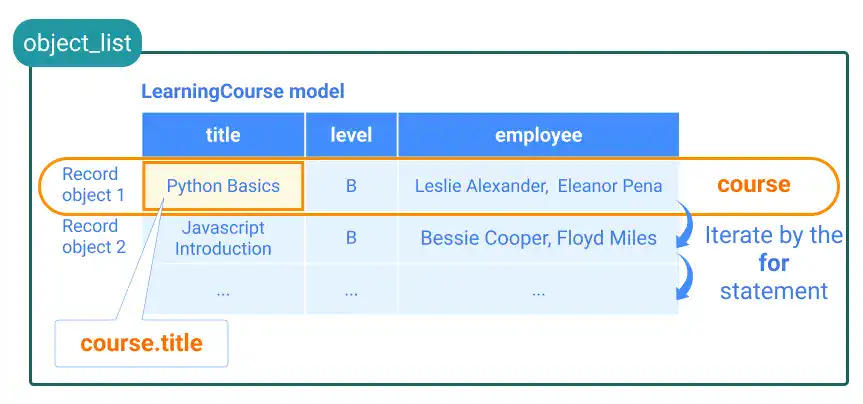
Practice
In this practice, we'll create a simple template for ListView by showing only one data field – the title of the learning course. Also, we'll explain how to change the title text to Uppercase using the filter
in this practice. In the end, we test a customized object_list
name defined in a previous section.
1. Edit course_list.html
Open the course_list.html file, add the yellow lines below, and save the file.
<h1>LIST Page</h1>
{% for course in object_list %}
<p> {{ course.title }}</p>
<hr>
{% endfor %}
2. Check the result in a browser
After saving the file, execute the runsever
command.
python manage.py runserver
Go to localhost:8000/employee-learning/course-list/
The URL is defined by the two urls.py files on the topic page URL Dispatcher for CRUD Views. You can see that the list of course titles is displayed.
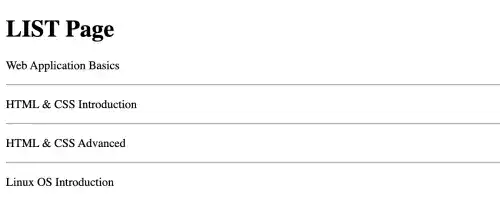
You might not have added dummy data yet if no data is displayed. Go to the Django admin page (localhost:8000/admin/) and add dummy data for the LearningCourse model.
3. Add the uppercase filter
Adding the uppercase
filter like the one below allows you to change the title name to uppercase.
:
{% for course in object_list %}
<p> {{ course.title|upper }}</p>
<hr>
{% endfor %}
After saving the HTML file, recheck the site. You'll see that the list is shown in uppercase.
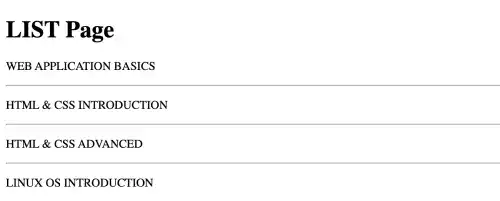
4. Check the user-friendly name for object_list
In CourseList, we set context_object_name = 'course_object_list'
. Check if it works by updating the code like the yellow code below.
:
{% for course in course_object_list %}
<p> {{ course.title|upper }}</p>
:
Go to localhost:8000/employee-learning/course-list/. You can confirm that the browser still shows the same results.
For the ListView in the Django official documentation, check this link Django documentation reference: ListView.