Create Index HTML

In the previous section, we created CRUD pages but haven't made a home page yet. Usually, index.html is used for the home page.
To create the home page, you need to update the urls.py files to redesign the URL structure, add a view for the home page and update template files.
Redesign URL structure (Update the urls.py files)
This exercise may be a good for understanding how to design URL patterns in the url.py files. In the current structure, the main url.py under the config directory is the first touch point when an HTTP response comes. Then, it includes the urlpatterns
written in url.py under the employee_learning app.
The main urls.py
First, you need to adjust the way employee_learning app's paths can be included. We used 'employee-learning
' as a part of the URL to make a clear contrast between test_app
and employee_learning
for an explanation purposes, but you can leave that part blank so that you can define the view of the index page in urls.py in the employee_learning
app.
After the steps above are completed, URLs of the pages for the employee_learning app become shorter as you don't need to use full paths of the directory tree.
To execute the change, update the file like in the example below.
:
urlpatterns = [
path('', include('employee_learning.urls')),
path('admin/', admin.site.urls),
path('test-app/', include('test_app.urls')),
# path('employee-learning/', include('employee_learning.urls')),
]
The app urls.py
Next, you need to define the path and view of the home page. As the home page should be accessible when a user accesses the domain itself, there is no need to add an additional path. For the view, use Index
, which will be defined in the next step.
from .views import ..., Index
:
urlpatterns = [
path('', Index.as_view(), name='index'),
path('course-list/', CourseList.as_view(), name='course_list'),
:
Create Index view
As each dynamic page should have a view, we need to make a view even for a simple home page.
Here is an example code. To use TemplateView, import it and define the template file (index.html). And add one extra context to show a date.
from django.views.generic import ListView, DetailView, CreateView, UpdateView, DeleteView, TemplateView
:
class Index(TemplateView):
template_name = 'index.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['today'] = datetime.date.today()
return context
Update Template files
Create the index.html file
First, you need to create a new HTML file for the home page. Create index.html under the templates directory and edit the file like shown below.
{% extends "base/base.html" %}
{% block body %}
<div class="container text-center">
<h1 class="text-center mt-5">Digital Training Registration</h1>
<p class="text-muted text-center mb-5">As of {{ today }}</p>
<a href="{% url 'course_list' %}" class="btn btn-primary m-3">View Registered Programs</a>
<a href="{% url 'course_create' %}" class="btn btn-primary m-3">Create a New Program</a>
</div>
{% endblock %}
Edit the navbar.html
To make a link to the home page on the brand logo in the navigation bar, edit the navbar.html file.
<a class="navbar-brand" href="{% url 'index'%}">Training Registration</a>
Go to http://localhost:8000/. Now, you won't see the yellow page anymore, and you can go to the home page instead.
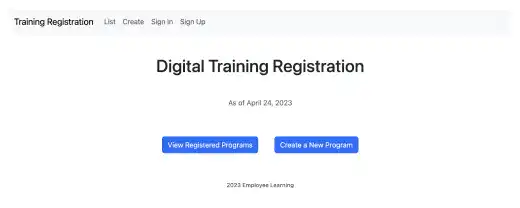