User Models

Django provides a built-in user model with predefined fields and methods, including the following fields.
username
first_name
last_name
email
password
groups
user_permissions
is_staff
is_active
is_superuser
last_login
date_joined
You may want to add or select the required fields to the app you are developing. There are two primary ways to do it.
- Extending the user model
- Substituting the user model
1. Extending the user model – Profile model with OneToOne relationship
In your app, you may want to add more fields on top of the Django built-in user model, such as a user icon, mobile number, and other user profiles. Unless you intend to delete or modify a user authentication method, extending the user model may be easier.
The approach is to create an extended model using OneToOneField
. Create a new model (e.g., the UserProfile model) with a One-To-One relationship with the built-in user model.
Practice 1
Objective:
Extending the User Model (User Profile)
In this practice, we'll demonstrate how to extend the built-in user model by making a new UserProfile model that has a One-To-One relationship with the built-in user model.
1. Create a new app
As the UserProfile model is not related to any of the models under the employee_learning app, it is better to create a new app by running the startapp
command. We use 'users' as an app name this time.
python manage.py startapp user_profile
2. Add the app in settings.py
As the app is new, add it under INSTALLED_APPS
in settings.py.
INSTALLED_APPS = [
:
'user_profile',
:
]
3. Add the UserProfile model in models.py
Open models.py under the new app, and add the code below.
from django.db import models
from django.contrib.auth.models import User
class UserProfile (models.Model):
user = models.OneToOneField(User, on_delete=models.CASCADE)
mobile = models.CharField(max_length=15, blank=True)
def __str__(self):
return self.user.username
4. Edit admin.py
To add the UserProfile model to the admin page, edit the admin.py file under the users directory.
from django.contrib import admin
from .models import UserProfile
admin.site.register(UserProfile)
5. Check the results
Run the makemigrations
and migrate
commands as we have created a new model. To see the outcome, execute the runserver
command.
python manage.py makemigrations user_profile
python manage.py migrate
python manage.py runserver
Go to localhost:8000/admin. You can see that the UserProfile model is registered under the new app. Using the same approach, you can extend the user model further.
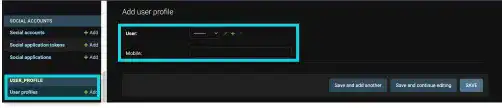
Substituting the user model – Creating a custom model using AbstractUser
If you want to have a more customized user model with specific fields, you need to build a custom model inheriting the built-in user model.
AbstractUser vs. AbstractBaseUser
There are two built-in user models that you can utilize – AbstractUser and AbstractBaseUser.
AbstractUser
As AbstractUser is designed based on AbstractBaseUser, more fields and attributes are available. If you want to make a custom user model quickly, inheriting AbstractUser is better. However, this approach may be less flexible as there may be fields that you may not want to include in your custom user model (e.g., first_name
and last_name
).
AbstractBaseUser
AbstractBaseUser provides only the core implementation of a user model. Using AbstractBaseUser, you can build a new custom user model almost from scratch. If you want to design your custom user model in a more flexible way, it is better to inherit AbstractBaseUser. However, you need to keep in mind that more code is required to implement a new custom user model.
Practice 2
Objective:
Substituting the User Model
In this practice, we'll demonstrate how to develop a custom user model by inheriting AbstractUser. As we have migrated the user model to the employee learning app, we'll generate a new project for this practice.
1. Start a new project
To create a new project, open a new window in VS Code. Create a new folder named 'custom_user', and open Terminal in VS Code.
Then, run the following commands to create a virtual environment and prepare for Django installation.
python3 -m venv venv
source venv/bin/activate
touch requirements.txt
Edit the requirements.txt file in VS Code by adding Django with version information.
Django==4.1.7
Execute pip
to install Django and run the startproject
command.
python -m pip install --upgrade pip
pip install -r requirements.txt
django-admin startproject config .
Run the runserver command.
python manage.py runserver
Check localhost:8000. If you can see the following screen, your project start is successful.
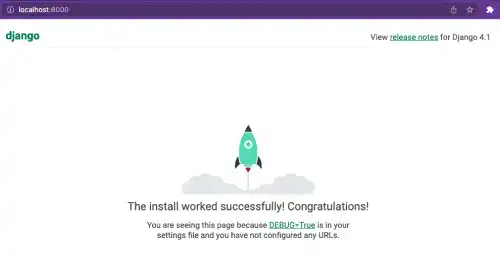
2. Create a new app and register it
Create a new app named 'user_model' for practice purposes.
python manage.py startapp user_model
Add the app in settings.py.
INSTALLED_APPS = [
:
'user_model',
]
3. Create a custom user model
Create the CustomUser model in models.py. Add the' mobile
' field to compare with the previous practice later.
from django.db import models
from django.contrib.auth.models import AbstractUser
class CustomUser(AbstractUser):
class Meta(AbstractUser.Meta):
db_table = 'custom_user'
mobile = models.CharField(max_length=15, blank=True)
4. Register the custom user model in settings.py
This is an important step. The Django built-in user model is currently used for the Django user authentication system (e.g., logging in to the Django admin page). By adding this setting, the custom model will be used for the authentication system.
AUTH_USER_MODEL = 'user_model.CustomUser'
5. Edit admin.py
To add the CustomUser model to the admin page, edit the admin.py file under the user_model directory.
from django.contrib import admin
from .models import CustomUser
admin.site.register(CustomUser)
6. Check the results
Run the makemigrations
and migrate
commands as we created a new model. As this is a new project, run the createsuperuser
command as well. Then, run the runserver
command.
python manage.py makemigrations users
python manage.py migrate
python manage.py createsuperuser
python manage.py runserver
Go to localhost:8000/admin. You can see that the custom user model is registered under USER_MODEL and that the mobile
field has been added.
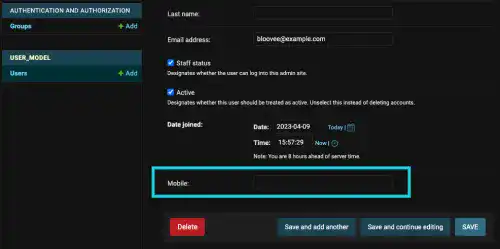
Note: Custom User Model
According to the Django official documentation, creating a custom user model is highly recommended.
![]()
If you’re starting a new project, it’s highly recommended to set up a custom user model, even if the default User model is sufficient for you. This model behaves identically to the default user model, but you’ll be able to customize it in the future if the need arises. Changing AUTH_USER_MODEL after you’ve created database tables is significantly more difficult since it affects foreign keys and many-to-many relationships, for example."
If you are planning to use a custom user model, it is better to avoid applying migrations until you complete the custom user model setup.