Start App

The django-admin startproject
creates only key files for the Django project configurations. To develop a Django application, you need to create key files used for actual application development. The startapp
command is used to create necessary files for Django application development.
The startapp command
The startapp
command is also executed through manage.py. When you run the command, you need to specify your application name. For example, run the command below to start an app named test_app.
python manage.py startapp test_app
When you run the command, you can see that many files are created under the new app directory.
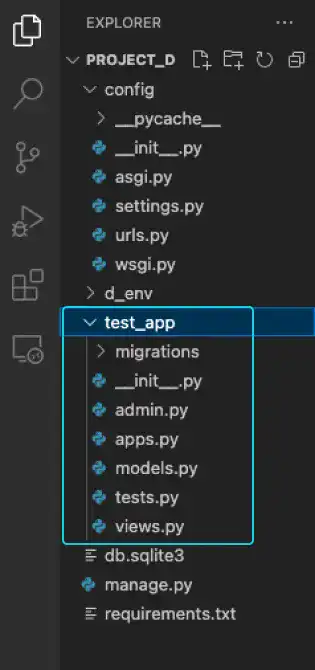
migrations directory
When you run the startapp
command, only the __init__.py file is created in this directory. This directory is used to store migration files. When you run the makemigration
command, a new migration file is created.
__init__.py
As explained in the Start Django Project section, this is a particular file for Python programming used to mark a directory as a Python package. Often, that file has no content. In regular use, you don't need to touch this file.
admin.py (Important)
This file is used when you customize the Django admin site using the data created by the app.
apps.py
This file is used to customize the configuration of the app. If you are a beginner in Django, you don't need to worry about this file.
models.py (Very Important)
This file is used to design the database of the app. In the file, you can describe the structures of the database, including its data field and data type settings. We'll explain how to use this file later.
tests.py
This file is used for writing and running tests for your Django application.
views.py (Very Important)
This file is one of the most critical files in the Django app development. You'll be frequently touching this file. Functions or classes for handling HTTP requests are designed through this file.
Register the new app
By only running the startapp
command, the Django project does not recognize the new app. To register the newly created app, you need to add the app name in the settings.py file.
In our example, add 'test_app' under the INSTALLED_APPS section of the settings.py file shown below. Do not forget to save the file to avoid making an error later on.
:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'test_app',
]
: