Create HTML Templates

When a Django app receives HTTP requests, the URL dispatcher dispatches them to functions or classes that are written in the views.py file to handle the requests. The views.py file focuses on logical responses. It doesn't handle visual representation for HTTP responses. To return the HTTP responses, you need HTML documents. In Django, the templates directory is used to save HTML documents linked to the views.py file. We'll explain how to make HTML templates for Django applications in this lesson. Details of views.py will be explained in the next section.
Create a 'templates' directory and an HTML document
As a default, the templates directory is not created yet. You need to create it to save HTML documents for the app. The best practice of the location of the templates directory is directly under the project directory. If you are using Mac, run the commands below from the project directory.
mkdir templates
cd templates
touch test.html
Or, you can create the directory and file from the left sidebar of VS Code.
Edit an HTML template
Let's create a classic message, "Hello World!" for the simple test purpose.
1. Select the test.html file in the VS Code.
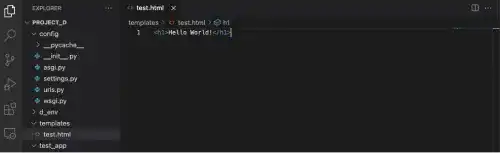
2. Edit the file.
Type the code below. As this template will be used to test the class-based view, describe it in the HTML file. We'll explain the class-based view in the next section.
<h1>Hello World! Class-based View Test</h1>
Register the 'templates' directory location in the settings.py
After creating the templates directory, you need to register the location of the directory in the settings.py file.
In the front part of the settings.py file, you can see that the path of BASE_DIR
is defined. BASE_DIR
is pointing to the project directory you created at the beginning of this chapter.
BASE_DIR
gives you flexibility to change the project directory path. Regardless of the project directory absolute path, you can use BASE_DIR
as the project directory path so that the file path stays the same even if you change your project directory location.
When you create a new directory right under the project directory, you can use BASE_DIR / 'sub-directory'
as the directory path. The sub-directory
part should be replaced with the actual directory name.
To add the templates directory you created under the project directory, add the yellow code part in the settings.py. The templates directory location should be written in the 'DIRS
' line in the TEMPLATES
section. In this case, first define the file path variable in the front section. Then, use the file path variable in the 'DIRS
' line.
Do not forget to save the settings.py file when you edit it.
from pathlib import Path
# Build paths inside the project like this: BASE_DIR / 'subdir'.
BASE_DIR = Path(__file__).resolve().parent.parent
# template directory path
TEMPLATE_DIR = BASE_DIR / 'templates'
:
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [TEMPLATE_DIR],
'APP_DIRS': True,