Django Models – ManyToManyField

To make a Many-To-Many relationship, Django uses ManyToManyField
. For the field, you need to specify a related model name. To connect the two tables using ManyToManyField, Django automatically creates the intermediary join table.
Practice
Objective:
Learn how to use ManyToManyField
In this case example, we'll create a new model LearningCourse, and connect it with the Employee model using a Many-To-Many relationship.
1. Add LearningCourse model in models.py
Create the LearningCourse model in the models.py file with ManyToManyField
to connect with the Employee model.
Add the model after the Employee model, as the LearningCourse model refers to the Employee model. Below is the code for the LearningCourse model.
The yellow lines below are the new code.
:
class Employee(models.Model):
:
class PersonalInfo(models.Model):
:
class LearningCourse (models.Model):
LEVEL=[('B','Basic'), ('I','Intermediate'), ('A','Advanced'),]
title = models.CharField(max_length=50, unique=True)
level = models.CharField(max_length=1, choices=LEVEL)
employee = models.ManyToManyField(Employee)
def __str__(self):
return self.title
2. Add LearningCourse model in admin.py
You need to add the new model in the admin.py file to reflect the model.
from .models import Employee
from .models import Division
from .models import PersonalInfo
from .models import LearningCourse
admin.site.register(Employee)
admin.site.register(Division)
admin.site.register(PersonalInfo)
admin.site.register(LearningCourse)
3. Execute the changes in the database and check the admin site
Run the three usual commands and go to the Django admin site.
python manage.py makemigrations employee_learning
python manage.py migrate
python manage.py runserver
You can see that the LearningCourse model is added and connected with the Employee model. As the relationship is Many-To-Many, you can select multiple employees.
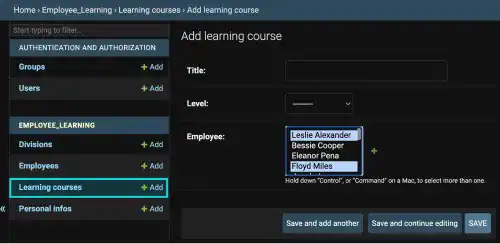