Create Views

Views are the key part of the Django application that defines how to handle HTTP requests. When a Django app receives HTTP requests, the URL dispatcher handles the request by calling specified views for each URL.
There are two types of views depending on how you write a view (logic):
- Function-based views
- Class-based views.
Function-based Views
Function-based views are views written with Python functions instead of classes.
The way to write a function-based view follows Python function syntax.
- Function names are usually written in snake_case (no uppercase letters. To connect words, use underscores).
- Use request as an argument of the function

The code below is an example of how to write a function-based view. The yellow parts of the code are added in this example.
In this case, we are using the HTTP response module. To import the module, add the second line.
- use 'function_view_test' as the function name.
- describe "Hello world! Function-based View Test" with the
<h1>
tag as an HTML response.
from django.shortcuts import render
from django.http import HttpResponse
def function_view_test(request):
return HttpResponse('<h1>Hello world! Function-based View Test</h1>')
Class-based Views
Django provides predesigned class-based views. You can customize the views through inheritance. Class-based views have been more widely used recently as they enable saving coding time.
The way to write a class-based view follows Python class syntax.
- Class name is usually written in PascalCase (Capitalize all words).
- Use the parent class name as an argument of the class to inherit the parent class
- Add a template file name saved under the templates directory
- If you use a model in the view, add a model name defined under the model.py
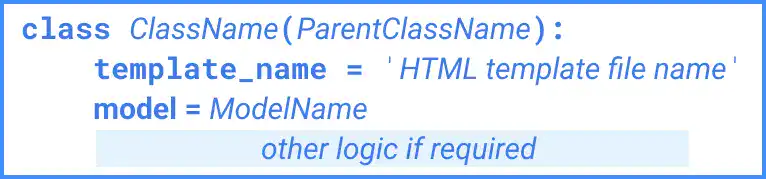
The code below is an example of how to write a class-based view. The yellow parts of the code are added in this example.
In this case, we are using TemplateView, which is one of the most simple predefined views. To import the view, add the third line.
To make clear that this example code is used for class-based view tests, use ''ClassViewTest" as the class name.
from django.shortcuts import render
from django.http import HttpResponse
from django.views.generic import TemplateView
def function_view_test(request):
return HttpResponse('<h1>Hello world! Function-based View Test</h1>')
class ClassViewTest(TemplateView):
template_name = 'test.html'
For more details about the class-based view, refer to Chapter 4.