Change Display Name of Record Objects

The records displayed in the Django admin site are usually Model Name + object (#), which is not user-friendly. For example, the data records of the Division model that we created on the previous page are shown below.
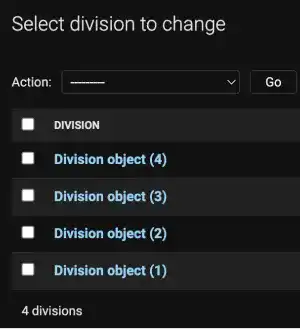
In Django, you can customize the record display name of each model by adding a simple code in models.py,
The __str__(self) method
The simplest way to change the record display name is by using one of the field names. For example, in the Division model, we can use field value in the div_name
field.
Edit the models.py file by adding the yellow line, as shown below.
class Division(models.Model):
div_name=models.CharField(max_length=25)
def __str__(self):
return self.div_name
Check the admin site after saving the models.py file. You can see that the record names have been changed.

Customize record names
By using f-string formatting, you can customize the record display name further. For example, if you want to show the ID and employee name as a record display name of the Employee model, edit models.py by adding the yellow line below.
class Employee(models.Model):
name=models.CharField(max_length=25)
def __str__(self):
return f'{self.id} : {self.name}'
Even though you don't create the id
field when you create a model, Django automatically creates the id
field, which is used as the model's primary key. You can call id
by 'self.id
'. self
is the Employee model class itself, and id
is an attribute of the class. 'self.name
' is the name
attribute of the Employee model class itself. The name
attribute is created when you design the model as a field name.
After saving the models.py file, go to the Django admin site. You can see that the records of the Employee model have been changed like shown below.
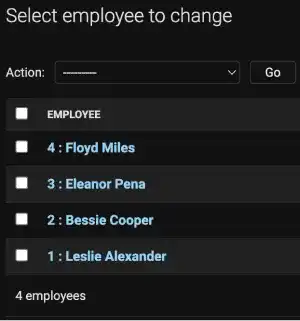