Makemigrations and Migrate

Once you have added a new model in the models.py file, you can start to create a database with two commands – makemigrations
and migrate
.
The makemigrations command
The makemigrations
command is used for creating a migration file, which is a design file used for creating a database. For example, if you want to create a migration file for the employee_learning app created in the previous section, run the command below.
python manage.py makemigrations employee_learning
You can see that the migration file 0001_initial.py has been created under the migrations directory.
Migrations for 'employee_learning':
employee_learning/migrations/0001_initial.py
- Create model Employee
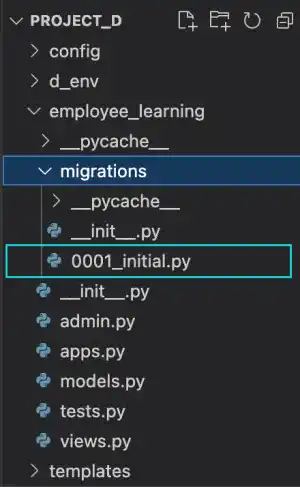
The migration files are created when you update the models.py file and run the makemigrations
command. The second time you run the makemigrations
command, you'll see that a new file named 0002_xxx.py is created. The xxx part of the filename is a simple description of key changes in the models.py file. Django captures the key changes and uses the information in the file name.
For example, add the Division model in the models.py file by adding the yellow line below and run the makemigrations
command.
from django.db import models
class Employee(models.Model):
name=models.CharField(max_length=25)
class Division(models.Model):
div_name=models.CharField(max_length=25)
python manage.py makemigrations employee_learning
You can see that the migration file 0002_division.py is created under the migrations directory.
Migrations for 'employee_learning':
employee_learning/migrations/0002_division.py
- Create model Division
The benefit of having two commands (makemigrations
and migrate
) in the database migration is that you can carefully manage the process. The makemigrations
step is especially helpful when you are updating the model after the database was populated with data.
For example, when new data fields are added to the models.py file, the command initiates the interactive mode and asks how to handle the data for new fields.
Note: App name for the makemigrations command
You can run the makemigrations
command without specifying an app name to create a migration file, but it is recommended to specify an app name to avoid potential future troubles.
For example, when you are making two apps in the same project, you may have two models.py files in each of the two apps: one with a completed model design and the other still in process of being designed. In that case, if you run the makemigrations
command without specifying an app name, Django will create migrations files for both apps. A migration file created by the incomplete models.py file may cause a problem in the database, which can be irreversible.
The migrate command
As explained in the previous chapter, the migrate command creates or updates a database using a migration file prepared by the makemigrations
command.
As we have already updated migration files, run the migrate
command again.
python manage.py migrate
You can see that two migration files were migrated into the database.
Operations to perform:
Apply all migrations: admin, auth, contenttypes, employee_learning, sessions, test_app
Running migrations:
Applying employee_learning.0001_initial... OK
Applying employee_learning.0002_division... OK