Main (List) Page Development

When a website has many pages, there is a page with a list of contents linking to each content page. The main page can be the same as the home page. For HTML and CSS practice purposes, we'll create different pages to explain different CSS techniques.
The image below is the target design of the main page example. In this example, we utilize components prepared in the previous chapter. The list layout is implemented using Flex Box.

In the practice section below, we'll explain how to implement the target design using the following steps.
- Add a background image
- Create a basic structure of the page with the following components
- Top bar
- Card (only one card component)
- Back button (to the home page)
- Footer
- Replicate the card component and complete the list design
Practice
Objective:
Create a list page with Flex Box
1. Create a new HTML file for the home page
- Create a copy of the index.html file and change the name to main-page.html.
- Change the
<title>
section to HTML & CSS Introduction Practice. - Also, delete the existing content of the
<body>
element that was created in the previous practice. - The code should look like the one below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!--Google Font-->
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Roboto:ital,wght@0,100;0,300;0,400;0,500;0,700;0,900;1,100;1,300;1,400;1,500;1,700;1,900&display=swap" rel="stylesheet">
<!--Custom CSS-->
<link rel="stylesheet" href="css/practice.css">
<link rel="stylesheet" href="css/component.css">
<title>HTML & CSS Introduction Practice</title>
</head>
<body>
</body>
</html>
2. Add a background image
To add a background image, we can use the technique explained in the previous chapter. For the main page, we use a green block wall image and add a translucent layer on top of it. You can choose a background image you like.
For this page, we use the <head>
section of the HTML file to set CSS as the CSS code is only applicable to this page.
This is the code for implementing the background image with a translucent layer on top.
<style>
body{
background: url(img/green-blocks.png) repeat center top/cover;
height: 100vh;
margin: 0;
padding: 0;
box-sizing: border-box;
}
.bg-cover-layer{
background-color:rgba(255,255,255,0.7);
height: 100vh;
margin: 0;
padding: 0;
}
</style>
</head>
<body>
<div class="bg-cover-layer">
</div>
</body>
At this point, this web page has a background only with no content, like shown in the image below.
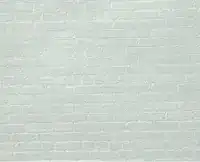
Tips: Live Server
When you develop code, keep running Live Server so that you can check the output in your browser in real-time.
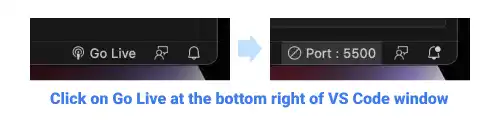
2. Create a basic structure of the page with components
We are adding the following components in this step:
- Top bar
- Card (only one card component)
- Back button (to the home page)
- Footer
To implement these components, add the code below within the <div>
element with the bg-cover-layer
class. As these components are the ones we have already prepared in the previous chapter, you don't need to add new CSS code.
<!--Top bar-->
<div class="top-bar" style="text-align: center; background-color: transparent;">
<a href="index.html" class="top-bar-icon">
<img src="img/html5.png">
<img src="img/css3.png">
</a>
</div>
<!--End top bar-->
<div style="display: flex; flex-wrap: wrap; justify-content: center">
<div class="card">
<a href="chapter1.html">
<div class="card-bg">
<span class="card-overlay-text">1. Overview</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 1.</small><br><b> Overview of Website Development</b></div>
<div class="card-icon"><img src="img/html5.png"><img src="img/css3.png"></div>
</div>
</a>
</div>
</div>
<br>
<div style="display: flex; justify-content: center; padding: 50px;">
<a href="index.html" class="btn-small">Back</a>
</div>
<!--footer-->
<footer style="height: 20px; background-color: transparent; position: initial;">
<p>HTML & CSS Introduction</p>
</footer>
<!--End footer-->
At this point, the structure of this page is set but it only has one card, like in the image below.
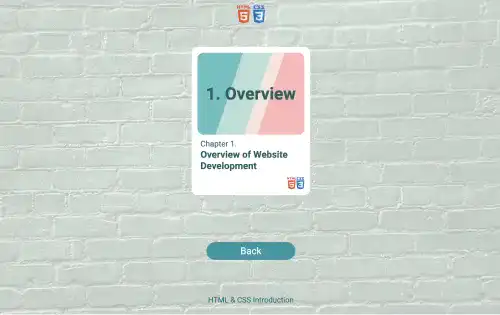
At this point, we haven't created a page for chapter 1. The link set on the card doesn't work. We'll make it work in the next chapter.
3. Replicate the card component and complete the list design
This step requires meticulous repetitive work. Copy the card component, and replicate it for Chapter 2 to Chapter 19 with some adjustments.
Adjust the following part for each chapter card:
- Link path (the
href
attribute part) - Card overlay text (use a short name for each chapter)
- Card title text (chapter number and title)
- Image icons (to indicate HTML and CSS topic coverage)
The code below is the complete set of code for the card component section. As the code is long, you can copy and paste it into your HTML document.
<div style="display: flex; flex-wrap: wrap; justify-content: center">
<div class="card">
<a href="chapter1.html">
<div class="card-bg">
<span class="card-overlay-text">1. Overview</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 1.</small><br><b> Overview of Website Development</b></div>
<div class="card-icon"><img src="img/html5.png"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter2.html">
<div class="card-bg">
<span class="card-overlay-text">2. Coding Preparation</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 2.</small><br><b> Preparing for Website Coding</b></div>
<div class="card-icon"><img src="img/html5.png"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter3.html">
<div class="card-bg">
<span class="card-overlay-text">3. HTML Basics</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 3.</small><br><b> HTML Basics</b></div>
<div class="card-icon"><img src="img/html5.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter4.html">
<div class="card-bg">
<span class="card-overlay-text">4. Links & Images</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 4.</small><br><b> HTML: Add Links and Images</b></div>
<div class="card-icon"><img src="img/html5.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter5.html">
<div class="card-bg">
<span class="card-overlay-text">5. Lists & Tables</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 5.</small><br><b> HTML: Create Lists and Tables</b></div>
<div class="card-icon"><img src="img/html5.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter6.html">
<div class="card-bg">
<span class="card-overlay-text">6. Forms</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 6.</small><br><b> HTML: Create Forms</b></div>
<div class="card-icon"><img src="img/html5.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter7.html">
<div class="card-bg">
<span class="card-overlay-text">7. HTML to CSS</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 7.</small><br><b> Bridging HTML and CSS</b></div>
<div class="card-icon"><img src="img/html5.png"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter8.html">
<div class="card-bg">
<span class="card-overlay-text">8. CSS Basics</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 8.</small><br><b> CSS Basics</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter9.html">
<div class="card-bg">
<span class="card-overlay-text">9. Web Design</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 9.</small><br><b> Web Design Basics</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter10.html">
<div class="card-bg">
<span class="card-overlay-text">10. Sizing & Spacing</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 10.</small><br><b> CSS: Sizing and Spacing</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter11.html">
<div class="card-bg">
<span class="card-overlay-text">11. Styling Text & Image</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 11.</small><br><b> CSS: Styling Text and Images</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter12.html">
<div class="card-bg">
<span class="card-overlay-text">12. Styling Backgrounds</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 12.</small><br><b> CSS: Styling Backgrounds</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter13.html">
<div class="card-bg">
<span class="card-overlay-text">13. Styling Borders</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 13.</small><br><b> CSS: Styling Borders and Drawing Lines</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter14.html">
<div class="card-bg">
<span class="card-overlay-text">14. Layout</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 14.</small><br><b> CSS: Layout – Key Concepts and Display Property</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter15.html">
<div class="card-bg">
<span class="card-overlay-text">15. Flex Box</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 15.</small><br><b> CSS: Layout – Flex Box</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter16.html">
<div class="card-bg">
<span class="card-overlay-text">16. Styling Lists</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 16.</small><br><b> CSS: Styling Lists</b></div>
<div class="card-icon"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter17.html">
<div class="card-bg">
<span class="card-overlay-text">17. Styling Components</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 17.</small><br><b> CSS: Styling Components</b></div>
<div class="card-icon"><img src="img/html5.png"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter18.html">
<div class="card-bg">
<span class="card-overlay-text">18. Create Websites</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 18.</small><br><b> Creating Websites</b></div>
<div class="card-icon"><img src="img/html5.png"><img src="img/css3.png"></div>
</div>
</a>
</div>
<div class="card">
<a href="chapter19.html">
<div class="card-bg">
<span class="card-overlay-text">19. Publishing</span>
</div>
<div class="card-body">
<div class="card-title"><small>Chapter 19.</small><br><b> Publishing Websites</b></div>
<div class="card-icon"><img src="img/html5.png"><img src="img/css3.png"></div>
</div>
</a>
</div>
</div>
Also, make a small adjustment in the CSS code for the card-overlay-text
class. As the card designed in the previous chapter had only one line of text, the text position looked okay. But when we use multiple lines, the text positions are not well aligned. To fix this, add text-align: center
to the card-overlay-text
class.
.card-overlay-text{
:
text-align: center;
}
4. Check the result with a browser using Live Server
If you are running Live Server, check your browser. You can see that the target design has been implemented.
If you are not running Live Server, click on the Go Live button at the bottom of VS Code. Your browser will open the home page.
You can also check the sample result here (Demo Site).