Shell Script

A shell script is a text file that contains a sequence of commands for a Linux or Unix operating system. In the command line, you can usually run only a single command. Using the shell script, you can run multiple commands as one operation without another human intervention during the process.
How to develop a shell script?
There are some rules to develop shell scripts.
File name
Add .sh at the end of the file name as a file extension.
Document structure
- Declaration: You need to specify which shell will be used for the shell script.
- Comment: You can add comments with
#
at the beginning of comments
Here is an example of creating a shell script using some commands discussed in the previous section.
Use vim to create a shell script file named test.sh.
vim test.sh
In the document, add a declaration and commands with comments like the one below. Press the i key to switch to the insert mode. Next, copy the code below and paste it into the file. After pasting the code, press the esc key followed by the : key and save the file by pressing the w + q keys. To learn how to use Vim, check Chapter 3. Vim Editor.
#!/bin/bash
# display product items
cat purchase_product.txt
# extract unique items and count duplicated items
sort purchase_product.txt | uniq -c
Add an execution permission
Usually, execution permission is not added when you create a file. In order to make the script executable, you need to add an execution permission to the file. To grant permission to the owner of the file, run the chmod u+x
command followed by the file path.
For the test.sh file, grant the execution permission to the owner user.
sudo chmod u+x test.sh
Run a shell script
To run a shell script, you just need to specify the file path of the shell script like the one below.
./test.sh
You'll see the original list of purchased products followed by unique product items with numbers of duplicated items.
Apple
Orange
Apple
Apple
Bananas
Apple
Grapes
Orange
Bananas
Grapes
Grapes
Bananas
Bananas
Apple
Grapes
5 Apple
4 Bananas
4 Grapes
2 Orange
Call another shell script from a shell script
You can call another shell script from a shell script. This is like creating a child process from a parent process.
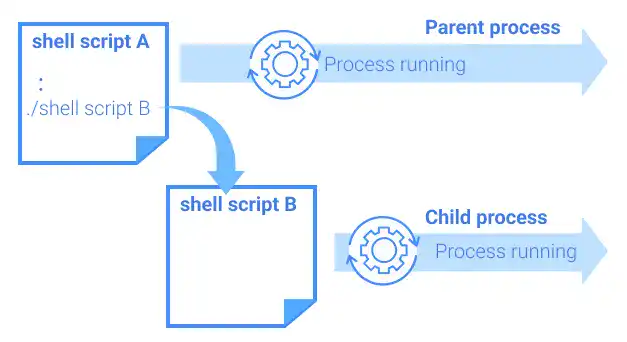
To call another shell script, you just need to add a shell script execution command to the shell script.
For example, create a new shell script file named test_child.sh and edit the file as shown below.
Note: the echo
command will be explained in the next section.
vim test_child.sh
#!/bin/bash
echo "----------------------------------------------"
echo "Unique Product List with # of Duplicated Items"
Also, copy the test.sh file and create a new file test_parent.sh. Then, edit the file to add a command to execute test_child.sh.
cp test.sh test_parent.sh
vim test_parent.sh
Add ./test_child.sh between the cat
command and the sort
command.
#!/bin/bash
# display product items
cat purchase_product.txt
./test_child.sh
# extract unique items and count duplicated items
sort purchase_product.txt | uniq -c
To execute the shell scripts, add permissions and run the test_parent.sh like shown below.
sudo chmod u+x test_parent.sh
sudo chmod u+x test_child.sh
./test_parent.sh
You can see that test_parent.sh successfully called test_child.sh.
Apple
Orange
Apple
Apple
Bananas
Apple
Grapes
Orange
Bananas
Grapes
Grapes
Bananas
Bananas
Apple
Grapes
----------------------------------------------
Unique Product List with # of Duplicated Items
5 Apple
4 Bananas
4 Grapes
2 Orange