Check Differences – git diff

git diff
is the command used when you want to know the differences between the Working Tree, the INDEX (Staging Area), and commit histories.
There are four types of differences you can get with the command.
- Difference between the Working Tree and INDEX: When you simply run
git diff
, you'll see this difference. - Difference between the Working Tree and one of the commits: To see this difference, you need to indicate a commit hash after
git diff
. - Difference between INDEX and HEAD (the latest commit): The
--cached
option allows you to see the difference between INDEX and one of the commit histories. When you don't specify a commit hash, the command gives you the difference between INDEX andHEAD
(the latest commit) - Difference between INDEX and a specific commit: When you see this difference, you need to specify a commit hash after the
--cached
option.
Practice
Developer A (Project Owner Role)
Objective:
Practice several git commands and test the git diff command
1. Open the practice HTML file
In this practice, we'll use the same practice file that was used in the previous chapter: git_practice.html.
The initial file content is shown below. First, confirm that the color status is blue
which we'll change later.
<!doctype html>
<html lang="en">
<head>
<style>
h1 {
color: blue;
font-size:80px
}
</style>
</head>
<body>
<h1>Hello World!</h1>
</body>
2. Edit the HTML file and make commits
In the HTML file, change the color property to green
as shown below. Keep the rest of the code unchanged and save the file.
h1 {
color: green;
font-size:80px
}
Then, make a new commit.
git commit -a -m "Modified commit"
[master 446fa13] Modified commit
1 file changed, 1 insertion(+), 1 deletion(-)[master 446fa13] Modified commit
1 file changed, 1 insertion(+), 1 deletion(-)
To check the commit status and commit hash, run the git log
command with the --oneline
option like below.
git log --oneline
The command line returns the following commit log with a short version of commit hash.
446fa13 (HEAD -> master) Modified commit
acc4aa4 (origin/master) added .gitignore file
651e510 the first commit
To create different statuses between the INDEX (Staging Area) and the latest commit (HEAD
), further change the color status to yellow
.
h1 {
color: yellow;
font-size:80px
}
After saving the file, stage the file by running the following command.
git add -A
Lastly, change the color status to red
and save the file to create a different status for the Working Tree.
h1 {
color:red;
font-size:80px
}
By this moment, the status of your git_practice.html file's commits, INDEX, and the Working Tree becomes the following. To simplify the illustration, we skipped the second commit "added .gitignore file", which is not used in this practice anyway.
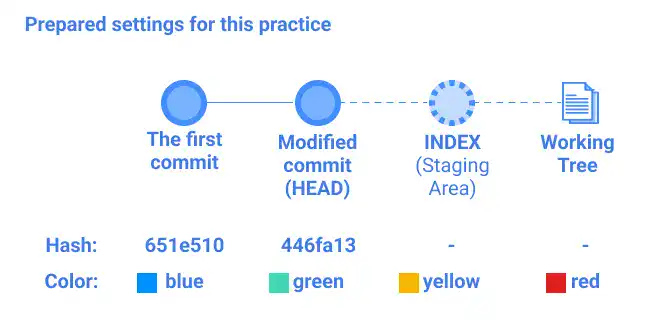
3. Check for differences
Using the information above, let's confirm the differences in several combinations.
Difference between the Working Tree and INDEX (Case 1)
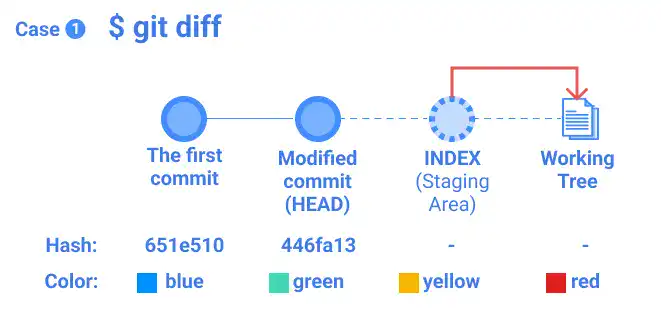
git diff
diff --git a/git_practice.html b/git_practice.html
index 9520b03..cd27dd6 100644
--- a/git_practice.html
+++ b/git_practice.html
@@ -3,7 +3,7 @@
<head>
<style>
h1 {
- color: yellow;
+ color: red;
font-size:80px
}
</style>
You can see a record showing that the color statuses are the following:
- The older color (INDEX) is
yellow
. - The newer color (Working Tree) is
red
.
Difference between the Working Tree and one of the commits (Case 2)
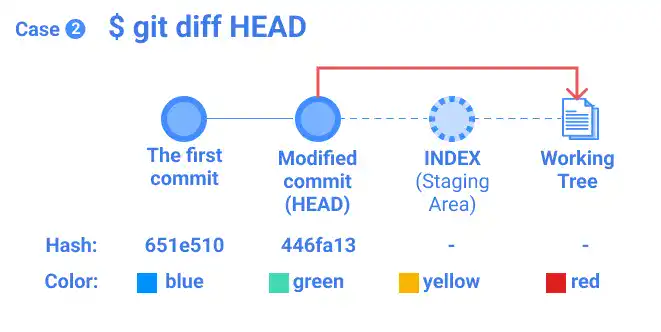
To check the difference between the Working Tree and HEAD
, run the command below.
git diff Head
Or
git diff 446fa13
446fa13
is the commit hash of HEAD
.
<style>
h1 {
- color: green;
+ color: red;
font-size:80px
}
</style>
You can see a record showing that the color statuses are the following:
- The older color (
HEAD
) isgreen
. - The newer color (Working Tree) is
red
.
Difference between INDEX and HEAD
(Case 3)
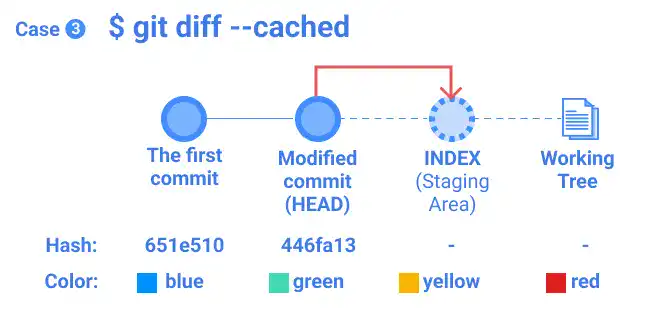
To check the difference between INDEX and HEAD
, run the command below.
git diff --cached
<style>
h1 {
- color: green;
+ color: yellow;
font-size:80px
}
</style>
You can see a record showing that the color statuses are the following:
- The older color (
HEAD
) isgreen
. - The newer color (INDEX) is
yellow
.
You can see a record showing that the color status has changed from green
to yellow
.
Difference between INDEX and a specific commit (Case 4)
![git diff visual explanations: Case 4 git diff --cached [commit hash] git diff visual explanations: Case 4 git diff --cached [commit hash]](https://static.d-libro.com/01-course-content-images/2041-10-Git-GitHub-Introduction/020-image-insert/git-diff-visual-explanations-case-4-git-diff-cached-commit-hash-id204110040810-img05.webp)
To check the difference between INDEX and the first commit, run the command below. 651e510
is the commit hash of the first commit.
git diff --cached 651e510
<style>
h1 {
- color: blue;
+ color: yellow;
font-size:80px
}
</style>
You can see a record showing that the color statuses are the following:
- The older color (commit
651e510
) isblue
. - The newer color (INDEX) is
yellow
.
Note: Check differences between branches
The git diff
command can be used for checking differences between branches. If you want to see the differences between Branch_1 and Branch_2, run the following command.
git diff [Branch_1]..[Branch_2]
The differences are shown after +
or -
. +
means that the lines of code were added in Branch_2 compared to Branch_1. -
means that the lines of code were deleted in Branch_2 compared to Branch_1.
+ code added
- code deleted
Branch operations will be explained in more detail in Chapter 5.