Z-Index to Manage Layers in CSS

Managing layers in CSS is crucial for achieving the desired visual hierarchy and layout on a webpage. The z-index
property plays a key role in determining how elements stack on top of each other. Understanding and applying z-index
effectively allows you to create visually appealing designs while maintaining control over how elements are layered. In this guide, we’ll explore how to manage CSS layers using the z-index
property, provide code examples, and introduce AI tools to help generate code quickly.
In this section, we’ll cover the following topics:
- Understanding CSS Layers and Layering Concepts
- Introduction to Z-Index in CSS
- Utilizing Z-Index with AI
Understanding CSS Layers and Layering Concepts
CSS layers define the order in which HTML elements appear visually on a page. Every element on a webpage exists on its own layer by default, and the stacking of these elements is determined by the CSS stacking context.
How CSS Layering Works
Layering in CSS follows a concept known as the stacking order. This order determines which elements are in front and which are behind. The natural flow of HTML elements from top to bottom already creates a basic layering structure, but to control more complex layouts, you need to define which elements should overlap and which should remain behind.
Elements are placed in stacking contexts, and by manipulating these contexts, you can control the layers. Each context is treated as a group, and all elements within it follow a stacking order relative to each other. The z-index
property is the primary method for controlling this order within a stacking context.
The Importance of Layering for Visual Design
Layering is essential for creating complex layouts and user interface components, such as modals, dropdown menus, and overlapping images. Without effective layer management, your design might not function properly, with important elements being hidden behind others. Mastering z-index
enables you to manage the visibility of each element on the page.
Introduction to Z-Index in CSS
The z-index property in CSS allows you to control the vertical stacking of elements. It’s used to manage which element should appear in front of or behind others. By assigning different z-index values, you can alter the stacking order.
What Is Z-Index in CSS?
The z-index property determines the stack level of an element within its stacking context. Elements with higher z-index values are placed above elements with lower z-index values, as long as they share the same stacking context. By default, all elements have a z-index of auto, meaning they stack according to the order they appear in the HTML.
Possible Values of Z-Index
- Auto:
auto
is the default value for z-index. When set to auto, the element follows the natural stacking order of the document. - Integer Values: You can assign any integer value to
z-index
, both positive and negative. A higherz-index
number will place the element on top of those with lowerz-index
values. - Maximum value: The maximum practical value for
z-index
is around2147483647
, as most browsers treatz-index
as a 32-bit signed integer. - Minimum value: The minimum value is around
-2147483648
. Negative values place elements behind others with higher values.
Note: In most cases, you won’t need to set z-index
values anywhere near these extremes. Typically, developers use small positive and negative numbers like z-index: 10
or z-index: -1
to manage stacking order effectively.
Meaning of Negative Z-Index
When an element is given a negative z-index
, it will appear behind other elements with a higher z-index
or the default stacking order. Negative z-index
values are often used when you want an element to sit beneath others but still within the same stacking context.
For example:
.element-behind {
position: relative;
z-index: -1; /* This element will be placed behind others with higher z-index values */
}
Default Stacking Order in CSS Layer
In CSS, the default stacking order is determined by the following criteria, starting from the lowest level:
- The background and borders of the root element.
- Non-positioned elements, in the order they appear in the HTML.
- Positioned elements (
absolute
,relative
,fixed
, orsticky
) withoutz-index
, in the order they appear in the HTML. - Positioned elements with a defined
z-index
.
Setting Z-Index to Control Layer CSS
To modify the stacking order using z-index
, you need to set the property on a positioned element.
Example:
<div class="box-1">Box 1</div>
<div class="box-2">Box 2</div>
<style>
.box-1 {
position: absolute;
z-index: 1;
width: 100px;
height: 100px;
background-color: red;
color: white;
}
.box-2 {
position: absolute;
z-index: 2;
width: 100px;
height: 100px;
background-color: blue;
top: 50px;
left: 50px;
color: white;
}
</style>
In this example, .box-2
will appear on top of .box-1
due to the higher z-index
value.
To view the result: Save the code as example-1.html
and example-1.css
in your folder. Open example-1.html
in your browser to see the blue box overlapping the red box.
Utilizing Z-Index with AI
This section will walk you through how to integrate AI to quickly generate the CSS and HTML code needed to manage layers with z-index
. With AI tools like ChatGPT, you can quickly produce code that matches your design goals, saving time and reducing errors.
Preparing for Practice Files
This course takes a hands-on approach, allowing you to apply the techniques covered in real-world scenarios. We'll be using a structured folder layout. Before proceeding with the examples, please ensure the following files are prepared:
/your-project-folder/
├── 04-12-z-index/ (<- sub-folder)
├── example-1.css
├── example-1.html
├── example-2.css
├── example-2.html
For your convenience, these files are also available on our GitHub repository. You can download the practice files to follow along with the case studies presented in this guide.
AI Case 1: Creating Overlapping Layers in CSS
In this case, we demonstrate how to create overlapping layers.
Sample AI prompt:
Create HTML and CSS code to demonstrate overlapping elements using z-index in two cases.
- Case 1: Use light blue for the first element and light green for the second element, with the light green element overlapping the light blue one.
- Case 2: Swap the layers so that the light blue element overlaps the light green one.
Use Flexbox to display both cases side by side.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Z-Index Overlapping Elements</title>
<link rel="stylesheet" href="example-1.css" />
</head>
<body>
<div class="container">
<!-- Case 1: Light green overlaps light blue -->
<div class="case case-1">
<div class="element element-1">Element 1</div>
<div class="element element-2">Element 2</div>
</div>
<!-- Case 2: Light blue overlaps light green -->
<div class="case case-2">
<div class="element element-1">Element 1</div>
<div class="element element-2">Element 2</div>
</div>
</div>
</body>
</html>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
.container {
display: flex;
justify-content: space-around;
}
.case {
position: relative;
width: 300px;
height: 200px;
border: 1px solid #ccc;
margin: 20px;
}
.element {
position: absolute;
width: 150px;
height: 150px;
top: 25px;
left: 25px;
}
/* Case 1 styles */
.case-1 .element-1 {
background-color: lightblue;
z-index: 1;
}
.case-1 .element-2 {
background-color: lightgreen;
top: 50px;
left: 50px;
z-index: 2;
}
/* Case 2 styles */
.case-2 .element-1 {
background-color: lightblue;
z-index: 2;
}
.case-2 .element-2 {
background-color: lightgreen;
top: 50px;
left: 50px;
z-index: 1;
}
Instructions to see the results:
- Save the code above in
example-1.html
andexample-1.css
in the04-12-z-index
folder. - Open
example-1.html
in your browser to view the blue box overlapping the red box.
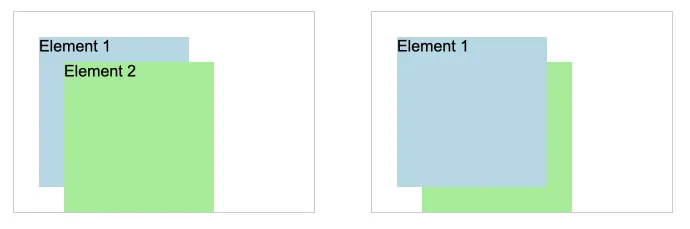
Visit this link to see how it looks in your web browser.
AI Case 2: Using Z-Index with Hover Effect
In this case, we demonstrate how to use the z-index property in CSS to control the stacking order of two overlapping boxes. When the user hovers over either box, the one behind moves to the front, showcasing the dynamic behavior of z-index in action.
Sample AI prompt:
Generate HTML and CSS code to create an example demonstrating the z-index effect using partially overlapping boxes with a hover effect. Include two boxes labeled “Box 1” and “Box 2”.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Z-Index Hover Effect with Overlapping Boxes</title>
<link rel="stylesheet" href="example-2.css" />
</head>
<body>
<p class="instruction">
Move the mouse over the light blue box to see the z-index effect.
</p>
<div class="container">
<div class="box box-1">Box 1</div>
<div class="box box-2">Box 2</div>
</div>
</body>
</html>
body {
font-family: Arial, sans-serif;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
background-color: #f4f4f9;
}
.container {
position: relative;
width: 300px;
height: 250px;
text-align: center;
}
.instruction {
position: absolute;
text-align: center;
top: 100px;
width: 100%;
color: #333;
font-size: 24px;
padding: 10px;
}
.box {
position: absolute;
width: 150px;
height: 150px;
display: flex;
align-items: center;
justify-content: center;
font-size: 20px;
color: white;
transition: all 0.3s ease;
}
.box-1 {
background-color: #6fa3ef;
top: 50px;
left: 25px;
z-index: 1;
}
.box-2 {
background-color: #7fc97f;
top: 75px;
left: 75px;
z-index: 2;
}
/* Hover effect: Bring the hovered box to the front */
.box:hover {
z-index: 3;
transform: scale(1.05); /* Optional: Slightly enlarge on hover */
}
Instructions to see the results:
- Save the code above in
example-2.html
andexample-2.css
in the04-12-z-index
folder. - Open
example-2.html
in your browser to view the blue box overlapping the red box.
Watch this video to see what it looks like.
Visit this link to see how it looks in your web browser.
Best Practices for Managing Layers with Z-Index in CSS
Mastering z-index in CSS allows you to control the stacking order of elements, creating visually structured layouts with layered effects. Here are some best practices for managing layers effectively:
- Understand Stacking Contexts: Ensure you know which elements establish a new stacking context (like those with
position
values or CSS transforms). Stacking contexts affect how z-index values are applied, so it’s essential to recognize them to avoid unexpected layer behaviors. - Use Integer Values Thoughtfully: Assign integer values to z-index thoughtfully, with small increments. Avoid excessively high values like
9999
; instead, use numbers like1
or10
to keep code maintainable and layering clear. - Apply z-index to Positioned Elements Only: Only elements with position values (
relative
,absolute
,fixed
, orsticky
) respect z-index values. Confirm that the element needing layering control has one of these positions applied. - Avoid Overlapping Layers Unnecessarily: Limit layering complexity by stacking only necessary elements. Too many overlapping layers can make code harder to debug and may affect performance.
- Test Across Browsers: Cross-browser testing helps ensure that z-index layers render consistently. Browsers may interpret stacking contexts differently, especially with complex layouts.
Following these practices will give you greater control over the visual order of elements on your webpage, allowing for cleaner, more maintainable CSS.