Display Property CSS with AI Prompt

The display property in CSS is essential for controlling the layout of elements on a web page. It specifies how elements are positioned and viewed, whether as inline, block, or within newer constructs like flexbox or grid layouts. This property is pivotal in web design as it governs the flow of HTML documents and influences how elements interact with each other and their parent containers. In this guide, we'll delve into the workings of the display property with code examples crafted using AI prompts.
In this section, we’ll cover the following topics:.
- Basic Display Values
- Flexbox in CSS
- Utilizing Display Property with AI Prompt
Basic Display Values
Understanding the fundamental display values in CSS is crucial for effectively manipulating element layout and positioning. Let's explore these values and see how AI can assist in experimenting with them.
Inline vs. Block vs. Inline-Block
- Inline: Elements set to
display: inline;
do not start on a new line and only take up as much width as necessary. This setting is ideal for smaller content pieces like words or images within a sentence. - Block: Elements with
display: block;
start on a new line and stretch out as wide as possible to the left and right edges of their container. This is useful for sections of content that require clear separation, such as paragraphs and divs. - Inline-Block: Combining traits of both inline and block, elements set to
display: inline-block;
flow like inline elements but maintain block characteristics like width and height. This property is particularly useful for creating a grid of boxes that align well but are part of the same line.
For a detailed explanation, explore this guide on Inline vs. Block vs. Inline-Block.
Display: None
Setting an element to display: none;
effectively removes it from the document flow. Unlike visibility, which merely hides the element but retains its space, display: none;
makes it as if the element does not exist, thus freeing up space.
For a detailed explanation, explore this guide on Display: None.
Flexbox in CSS
Flexbox is a layout model that provides an efficient way to distribute space and align content flexibly within a container. Whether you are dealing with a complex application or a simple layout, Flexbox offers a powerful toolkit for managing layout with minimal effort.
Managing Flex Item positions
Flex Direction
The flex-direction
property determines the main axis along which flex items are laid out. You can choose between row
, column
, row-reverse
, or column-reverse
, depending on whether you want items arranged horizontally or vertically and in what order.
Main Axis vs. Cross Axis
The main axis is determined by the flex-direction
property, and the cross axis is perpendicular to the main axis. Understanding these axes helps when configuring layout alignment and spacing.
Flex Wrap
The flex-wrap
property allows flex items to wrap onto multiple lines when they overflow the flex container. By default, flexbox layouts are single-line, but flex-wrap: wrap;
will enable wrapping.
Justify Content
This property aligns flex items along the main axis. Options like space-between
, space-around
, and center
control how items are distributed within the container.
Align Items
This property aligns flex items along the cross axis, controlling the vertical alignment in a row
layout or horizontal alignment in a column
layout.
Align Content
This property manages the space between rows in a multi-line flex container, similar to how justify-content
works on a single line.
Align Self
align-self
allows overriding the alignment of individual flex items along the cross axis. This is useful when one item needs to behave differently from the others in the same flex container.
Adjust Flex Item Size
Flex Grow
The flex-grow
property allows a flex item to grow in relation to others, filling the available space in the container.
Flex Shrink
The flex-shrink
property specifies how much a flex item should shrink relative to others in the same container when space is limited.
Flex Basis
This property sets the initial size of a flex item before extra space is distributed or the item is allowed to grow or shrink.
Advanced Flexbox Approaches
Margin: Auto with Flexbox
margin: auto;
is a powerful tool in Flexbox layouts for centering items along the main axis. It adjusts the margins to evenly distribute space around an item, centering it within its container.
Inline Flex
display: inline-flex;
allows the flex container to behave like an inline element, flowing within a line of text. This is useful for when you need Flexbox functionality but want to keep elements in line with surrounding content.
Nested Flexbox Layouts
Nesting flexbox layouts allows for more complex structures, such as media objects with text and images, making it easier to create flexible, responsive designs.
To learn more about Flexbox, explore this guide on CSS: Layout – FlexBox.
Display Grid in CSS
The CSS Grid Layout is a powerful layout system that provides a two-dimensional approach to arranging elements, allowing both rows and columns to be designed in a grid-like format. It simplifies the process of creating complex layouts, making it easy to place items anywhere in the grid and control their size and spacing.
Grid Basics
CSS Grid operates using a parent element, known as the grid container, and its child elements, which are the grid items. The grid container defines the structure, while the grid items are arranged according to the defined grid layout.
Defining a Grid
You can define a grid using the display: grid;
property on a container.
Customizing Grid Layout
CSS Grid provides various properties to control grid item positioning, spanning, and sizing:
- grid-column and grid-row: These properties allow you to control how many columns or rows a grid item should span.
- gap: This defines the spacing between grid items.
- grid-auto-flow: Controls how auto-placed items are inserted into the grid.
Aligning Grid Items
Just like Flexbox, you can align grid items using properties like justify-items
, align-items
, justify-content
, and align-content
to control how items are aligned within the grid.
We'll explore Display Grid in greater detail later in Chapter 5.
Other Display Values: Table, Table-Cell, List-Item
Display properties can be used to define other types of elements, such as tables, table cells, and list items. These values allow for more specialized layouts, mimicking the behavior of HTML elements like tables or lists without explicitly using them.
display: table
The display: table
property allows you to create a container that behaves like an HTML <table>
element. It’s often used when you need to create table-like structures for aligning content without using actual table markup.
This setup behaves similarly to a standard HTML table but provides more flexibility for responsive designs.
display: table-cell
The display: table-cell
property mimics the behavior of a <td>
element. You can use this in combination with table
and table-row
to create grid-like layouts.
display: list-item
The display: list-item
property makes an element behave like a <li>
(list item) element, often used within <ul>
or <ol>
tags. It adds bullet points or numbering to the element when used within a list container.
Utilizing Display Property with AI Prompt
To effectively leverage the power of CSS display properties, we can use AI-driven code generation to make experimenting with different layouts easier and more efficient. By issuing simple AI prompts, we can generate CSS code that adapts layouts dynamically and visually.
In the following use cases, you’ll learn how to apply AI prompts to adjust button layouts, hide elements, and utilize Flexbox for different layout designs.
Preparing for Practice Files
This course takes a hands-on approach, allowing you to apply the techniques covered in real-world scenarios. We'll be using a structured folder layout. Before proceeding with the examples, please ensure the following files are prepared:
/your-project-folder/
├── 02-03-display-property/ (<- sub-folder)
├── example-1.css
├── example-1.html
├── example-2.css
├── example-2.html
├── example-3.css
├── example-3.html
├── original.css
├── original.html
Original Code
Before we modify the layout, here’s the original HTML and CSS code that we'll start with.
Copy the code below into original.html
and original.css
.
HTML (original.html):
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="original.css" />
</head>
<body>
<button class="btn-1">Button 1</button>
<button class="btn-2">Button 2</button>
<button class="btn-3">Button 3</button>
</body>
</html>
CSS (original.css):
button {
display: block;
padding: 10px;
margin: 5px;
}
.btn-1 {
background-color: lightblue;
}
.btn-2 {
background-color: lightgreen;
}
.btn-3 {
background-color: lightcoral;
}
Visit this link to see how the original HTML document looks in your web browser.
For your convenience, these files are also available on our GitHub repository. You can download the practice files to follow along with the case studies presented in this guide.
AI Case 1: Utilizing Inline Block to Adjust Button Layout
In this case study, we will modify the layout of the buttons to display them horizontally instead of vertically. This change demonstrates how using inline-block
can adjust element flow in a webpage.
Sample AI prompt:
Create CSS code to adjust the button layout to be horizontal, with buttons appearing next to each other.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="example-1.css" />
</head>
<body>
<button class="btn-1">Button 1</button>
<button class="btn-2">Button 2</button>
<button class="btn-3">Button 3</button>
</body>
</html>
button {
display: inline-block;
padding: 10px;
margin: 5px;
}
.btn-1 {
background-color: lightblue;
}
.btn-2 {
background-color: lightgreen;
}
.btn-3 {
background-color: lightcoral;
}
Instructions to see the results:
- Copy the original HTML and CSS from
original.html
andoriginal.css
intoexample-1.html
andexample-1.css
, respectively. - Update the CSS file link path in
example-1.html
- Update the CSS code in
example-1.css
with the CSS code above. - Open
example-1.html
in your browser to see the buttons appear in a horizontal line.
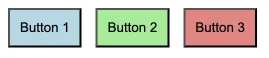
Visit this link to see how it looks in your web browser.
AI Case 2: Utilizing Display: None Property
In this case study, we will hide the second button while keeping the first and third buttons visible. This demonstrates how the display: none;
property can be used to completely hide an element from the page layout.
Sample AI prompt:
Create CSS code to hide the second button in the layout.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="example-2.css" />
</head>
<body>
<button class="btn-1">Button 1</button>
<button class="btn-2">Button 2</button>
<button class="btn-3">Button 3</button>
</body>
</html>
/* example-2.css */
button {
display: inline-block;
padding: 10px;
margin: 5px;
}
.btn-1 {
background-color: lightblue;
}
.btn-2 {
background-color: lightgreen;
display: none;
}
.btn-3 {
background-color: lightcoral;
}
Instructions to see the results:
- Copy the content from
example-1.html
andexample-1.css
intoexample-2.html
andexample-2.css
. - Update the CSS file link path in
example-2.html
- Update the CSS code in
example-2.css
with the CSS code above. - Open
example-2.html
in your browser to see the second button hidden while the first and third buttons remain in a horizontal layout.
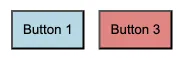
Visit this link to see how it looks in your web browser.
AI Case 3: Utilizing Flexbox – Flex Direction and Align Items
In this case study, we will align the buttons vertically within the viewport and distribute buttons 1 and 3 horizontally. This demonstrates how Flexbox properties such as justify-content
and align-items
can be used to center and distribute elements effectively.
Sample AI prompt:
Create CSS code to place the buttons in the center vertically and distribute button 1 and button 3 horizontally.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="example-3.css" />
</head>
<body>
<button class="btn-1">Button 1</button>
<button class="btn-2">Button 2</button>
<button class="btn-3">Button 3</button>
</body>
</html>
/* example-3.css */
button {
display: inline-block;
padding: 10px;
margin: 5px;
}
.btn-1 {
background-color: lightblue;
}
.btn-2 {
background-color: lightgreen;
display: none;
}
.btn-3 {
background-color: lightcoral;
}
body {
display: flex;
justify-content: space-between;
align-items: center;
height: 100vh;
}
Instructions to see the results:
- Copy the content from
example-2.html
andexample-2.css
intoexample-3.html
andexample-3.css
. - Update the CSS file link path in
example-3.html
- Update the CSS code in
example-3.css
by adding the code above. - Open
example-3.html
in your browser to see buttons 1 and 3 distributed horizontally while vertically centered within the viewport.
Visit this link to see how it looks in your web browser.
In this guide, we explored how to use CSS display properties, such as inline-block
, display: none
, and Flexbox layouts, to control the arrangement of elements on a webpage. By progressively modifying the layout using AI prompts, you learned how to experiment with different display properties and enhance your web design skills.
To learn more about display property, explore this guide on CSS: Layout – Key Concepts and Display Property.
Best Practices for Using CSS Display Properties
CSS display properties are essential for building responsive and well-organized web layouts. By focusing on core HTML and CSS techniques, you can achieve clean, accessible designs that enhance the user experience across devices. Here are some best practices for effectively using CSS display properties in your projects.
- Use Display Types Appropriately: Apply the basic display values (
inline
,block
,inline-block
) based on the element's function. Useblock
for full-width elements like sections or paragraphs,inline
for small elements within text, andinline-block
for items that need inline positioning but custom dimensions (like navigation links). - Master Flexbox for Simple Layouts: Flexbox is ideal for single-axis layouts, such as horizontally or vertically aligned sections. Use properties like
flex-direction
to set the main axis andjustify-content
to manage item spacing within a container. Flexbox provides alignment flexibility that adapts well across devices without complex code. - Use display: none Thoughtfully: Hiding elements with
display: none
can be useful, but consider accessibility. Make sure hidden elements are not essential for users or screen readers, and avoid hiding crucial navigation items, which can impact both usability and SEO. - Maintain Consistent Spacing and Alignment: Apply consistent padding, margins, and alignment across elements to create a clean and harmonious layout. Using properties like
margin: auto
andtext-align
helps align content effectively, making the design visually balanced and user-friendly.
Following these best practices will help you create clear, accessible, and adaptable web layouts. By mastering CSS display properties in tandem with well-structured HTML, you can achieve clean, scalable designs that enhance functionality and visual appeal.