Variables, Variable Declaration, and Variable Names in JavaScript

Variables form the backbone of programming, enabling developers to store, retrieve, and manipulate data. In JavaScript, mastering variables involves understanding their declaration, usage, and proper naming conventions. This guide will walk you through everything from the basics of variables to advanced practices, making your code efficient and readable.
In this section, we’ll cover the following topics.
- What Are Variables?
- How to Declare Variables in JavaScript
- Rules for JavaScript Variable Names
- Best Practices for Declaring Variables
What Are Variables?
Variables are fundamental to any programming language, allowing programs to handle dynamic data. In JavaScript, variables act as named containers that store values which can be retrieved or updated during execution. Without variables, creating meaningful interactions in web development would be nearly impossible.
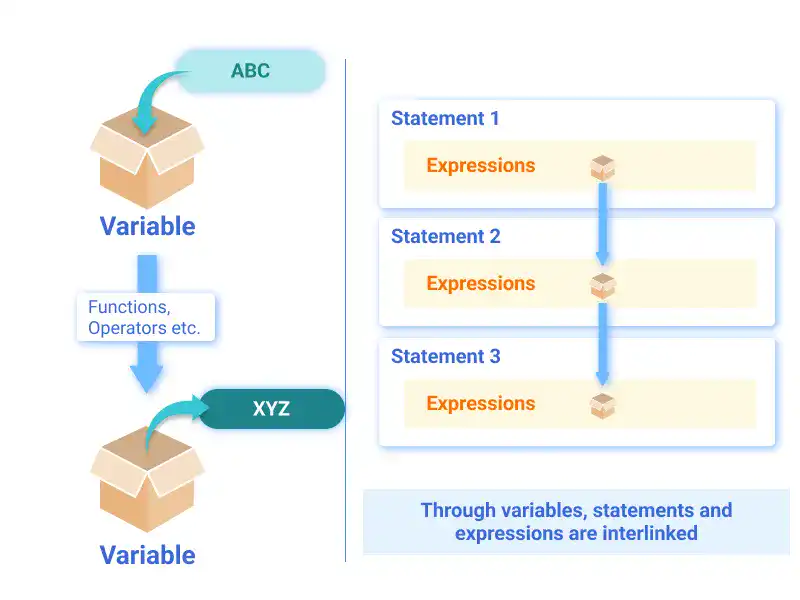
For example, in the code snippet below, the variable box
stores elements from a webpage:
let box = document.querySelectorAll(".grid-item");
for (let i = 0; i < box.length; i++) {
box[i].addEventListener("mouseover", mouseoverFunc);
function mouseoverFunc() {
box[i].style.backgroundColor = "#006e78";
}
box[i].addEventListener("mouseout", mouseoutFunc);
function mouseoutFunc() {
box[i].style.backgroundColor = "lightblue";
}
}
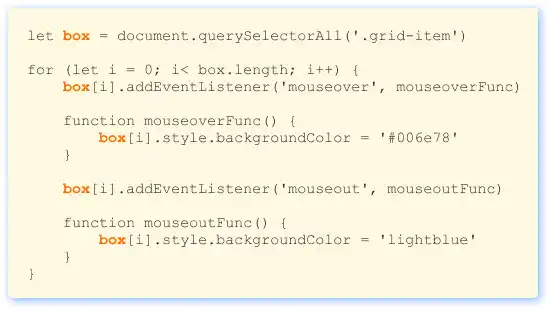
Here, box
holds a list of elements with the class grid-item
, and events like mouseover
and mouseout
trigger changes in their appearance.
How to Declare Variables in JavaScript
In JavaScript, variables can be declared using let
, const
, or the older var
. Each has specific use cases, making it essential to understand their differences.
Syntax for Variable Declaration - let, const, and var
let
: Best for block-scoped variables that need to change.const
: Use for constants or variables that won't be reassigned.var
: Older syntax, mostly replaced bylet
andconst
due to its function scope and lack of block scope.
let age = 25; // block-scoped, can be updated
const name = "Alice"; // block-scoped, immutable
var isStudent = true; // function-scoped, legacy use
Variables vs. Constants
While variables can be updated or reassigned, constants (const
) are immutable once declared. Use constants when the value should remain fixed, such as configuration settings or mathematical constants like pi
.
Use Variables (let
):
- When the value is expected to change, such as:
- User inputs.
- Iterators in loops.
- Temporary values that are updated during execution.
Use Constants (const
):
- For values that do not change throughout the program, such as:
- Mathematical constants (e.g.,
pi
,gravity
). - Configuration settings (e.g.,
MAX_USERS
). - API endpoints (e.g.,
BASE_URL
).
- Mathematical constants (e.g.,
- This makes your intentions clear to other developers, signaling that the value is meant to remain constant.
Block Scope vs. Function Scope
Understanding the difference between block scope and function scope is essential for writing efficient and predictable JavaScript code. Let’s break down these two concepts to clarify how they work and when to use them.
(We’ll explore variable scope in detail in Chapter 5, along with comprehensive explanations on functions. For now, we’ll briefly introduce the topic to highlight the key differences between let
, const
, and var
.)
Block Scope
Block scope refers to the visibility and lifetime of variables declared inside a block, which is any code enclosed within {}
(curly braces). Variables declared with let
or const
are confined to the block where they are defined, making block scope a safer and more predictable scoping method.
Example:
if (true) {
let blockScopedVar = "I exist only in this block!";
const blockScopedConst = "Me too!";
console.log(blockScopedVar); // Accessible here
}
console.log(blockScopedVar); // Error: blockScopedVar is not defined
Key Features of Block Scope:
- Local to the Block: Variables are accessible only within the block where they are declared.
- No Variable Leakage: Prevents variables from "leaking" outside their block, reducing the risk of naming conflicts.
- Modern Syntax: Introduced with
let
andconst
in ES6, making JavaScript code more modular and maintainable.
Function Scope
Function scope pertains to variables declared inside a function. These variables are accessible throughout the entire function, regardless of block boundaries within that function. Variables declared with var
use function scope, which can sometimes lead to unintended behavior.
Example:
function functionScopeExample() {
if (true) {
var functionScopedVar = "I belong to the function!";
}
console.log(functionScopedVar); // Accessible here because var is function-scoped
}
functionScopeExample();
console.log(functionScopedVar); // Error: functionScopedVar is not defined outside the function
Key Features of Function Scope:
- Local to the Function: Variables are accessible anywhere within the function, even outside nested blocks.
- Legacy Behavior: Function scope applies to variables declared with
var
, a pre-ES6 (ECMAScript 6) syntax.
Updating and Reassigning Variables
Understanding how variables can be updated or reassigned is critical for managing data in JavaScript. Variables behave differently depending on whether they are declared with let
, var
, or const
.
Reassigning Variables Declared with let or var
Variables declared with let or var can be reassigned to hold new values after their initial assignment. This makes them ideal for storing dynamic data that changes over time.
Example:
let score = 10; // Declare and assign a value
console.log(score); // Output: 10
score = 20; // Reassign to a new value
console.log(score); // Output: 20
Reassigning Constants Declared with const
Constants (const
) are different because they are immutable in terms of reassignment. Once a constant is declared and assigned a value, it cannot be reassigned to a new value.
Example:
const MAX_USERS = 100; // Declare and assign a value
console.log(MAX_USERS); // Output: 100
// Attempt to reassign
MAX_USERS = 200; // Error: Assignment to constant variable
Rules for JavaScript Variable Names
Naming variables in JavaScript requires adherence to specific rules to ensure clarity and avoid errors.
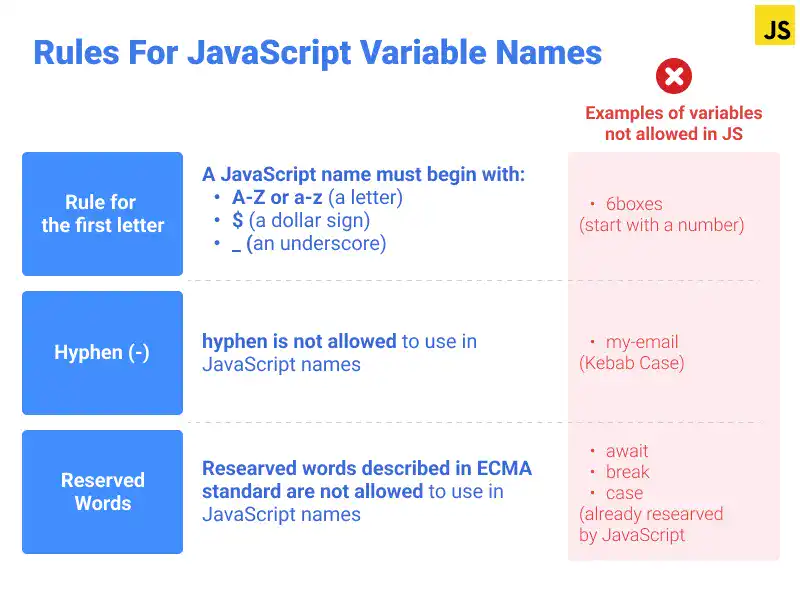
Rule for the First Letter
Variable names must begin with a letter, underscore (_
), or dollar sign ($
). Numbers can appear later but not at the start.
let userName = 'John'; // Valid
let _temp = 42; // Valid
let $price = 9.99; // Valid
let 1stPlace = 'Gold'; // Invalid
Hyphens
Hyphens (-
) are not allowed in variable names. Instead, use camelCase, PascalCase, or snake_case for readability.
let first-name = 'Alice'; // Invalid
let firstName = 'Alice'; // Valid (camelCase)
Reserved Words
Avoid using JavaScript reserved words (e.g., class
, var
, function
) as variable names. We’ll explore reserved words in detail later in this chapter.
Best Practices for Declaring Variables
Declaring variables effectively ensures your code remains clear and error-free. Follow these best practices:
- Use Descriptive Names: Variables should indicate their purpose. For example, use
userAge
instead ofx
. - Choose the Right Keyword: Use
const
by default andlet
when reassigning. Avoidvar
. - Avoid Global Variables: Minimize the use of globally scoped variables to prevent conflicts.
- Initialize Variables: Always assign an initial value to avoid unexpected behavior.
Closing note: Adopting these practices makes your code more readable, maintainable, and less prone to bugs.
Reference links: