Statements and Expressions in JavaScript

JavaScript is a fundamental programming language for building dynamic and interactive websites. To create robust and efficient code, understanding the distinction between statements and expressions is crucial. These two concepts form the foundation of JavaScript, allowing developers to structure logic, control flow, and computations effectively. In this guide, we will explore their roles, types, and applications with practical examples.
In this section, we’ll cover the following topics:
- What Are Statements in JavaScript?
- What Are Expressions in JavaScript?
- JavaScript Code Anatomy
What Are Statements in JavaScript?
A statement in JavaScript is a complete unit of code that performs a specific action or instruction. Statements execute sequentially to implement the desired behavior or logic within a program. They may include expressions, which evaluate values necessary for the statement to work.
For example:
let x = 10; // This is a variable declaration statement.
if (x > 5) {
console.log("x is greater than 5"); // Output statement inside a control flow.
}
Types of Statements
Here are the common types of statements in JavaScript:
Variable Declarations
Variable declarations define variables that can store data:
let name = "Alice"; // Declares and assigns a value to a variable.
Function Declarations
Functions are reusable blocks of code:
function greet() {
console.log("Hello!");
}
Conditional Statements
Control statements (e.g., if, switch) guide the program based on conditions:
if (x > 0) {
console.log("Positive number");
} else {
console.log("Non-positive number");
}
Loop Statements
Loops (e.g., for, while) execute blocks of code repeatedly under specific conditions:
for (let i = 0; i < 5; i++) {
console.log(i);
}
What Are Expressions in JavaScript?
An expression is a fragment of code that evaluates to a value. It can include variables, literals, operators, and function calls. Unlike statements, expressions do not perform actions directly but instead produce results that can be used within statements.
For example:
5 + 3; // An arithmetic expression that evaluates to 8.
"x" + "y"; // A string expression that evaluates to "xy".
Types of Expressions
Arithmetic Expressions
Perform mathematical operations:
10 - 3; // Evaluates to 7.
String Expressions (+ Operator)
Concatenate strings:
"Hello" + " " + "World"; // Evaluates to "Hello World".
Logical Expressions
Use logical operators to return Boolean values:
true || false; // Evaluates to true.
Function Expressions
Define anonymous functions within variable declarations:
const square = function (x) {
return x * x;
}; // The entire function is an expression.
JavaScript Code Anatomy
Understanding the anatomy of JavaScript code requires recognizing how statements and expressions work together. Below is an example demonstrating these concepts:
let box = document.querySelectorAll(".grid-item");
for (let i = 0; i < box.length; i++) {
box[i].addEventListener("mouseover", mouseoverFunc);
function mouseoverFunc() {
box[i].style.backgroundColor = "#006e78";
}
box[i].addEventListener("mouseout", mouseoutFunc);
function mouseoutFunc() {
box[i].style.backgroundColor = "lightblue";
}
}
Code Explanation
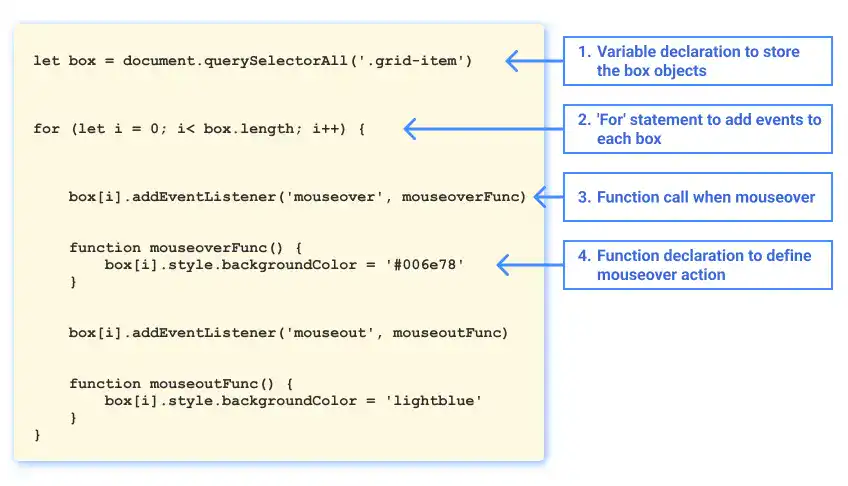
-
Variable Declaration
let box = document.querySelectorAll('.grid-item');
This statement declares thebox
variable and assigns it a collection of HTML elements. -
Loop Statement
for (let i = 0; i < box.length; i++) {}
Afor
loop iterates over thebox
elements, ensuring each one gets the specified behavior. -
Event Listener for Mouseover
box[i].addEventListener('mouseover', mouseoverFunc);
This adds amouseover
event listener to each box, calling themouseoverFunc
function when triggered. - Defining Functions
-
mouseoverFunc
: Changes the background color to a darker green. -
mouseoutFunc
: Restores the background color to light blue.
This example showcases how statements (e.g., variable declarations, loops) and
expressions (e.g., box.length
, i < box.length
)
integrate seamlessly to create dynamic functionality.
By mastering statements and expressions in JavaScript, you can write code that is not only efficient but also easier to debug and maintain. Each concept is a stepping stone in your journey toward becoming a proficient JavaScript developer.
Reference links:
FAQ: Statements and Expressions in JavaScript
What are statements in JavaScript?
A statement in JavaScript is a complete unit of code that performs a specific action or instruction. Statements execute sequentially to implement the desired behavior or logic within a program. They may include expressions, which evaluate values necessary for the statement to work.
What are expressions in JavaScript?
An expression is a fragment of code that evaluates to a value. It can include variables, literals, operators, and function calls. Unlike statements, expressions do not perform actions directly but instead produce results that can be used within statements.
What are the common types of statements in JavaScript?
Common types of statements in JavaScript include:
- Variable Declarations: Define variables that can store data.
- Function Declarations: Reusable blocks of code.
- Conditional Statements: Control statements (e.g., if, switch) guide the program based on conditions.
- Loop Statements: Loops (e.g., for, while) execute blocks of code repeatedly under specific conditions.
What are the common types of expressions in JavaScript?
Common types of expressions in JavaScript include:
- Arithmetic Expressions: Perform mathematical operations.
- String Expressions: Concatenate strings using the + operator.
- Logical Expressions: Use logical operators to return Boolean values.
- Function Expressions: Define anonymous functions within variable declarations.
How do statements and expressions work together in JavaScript code?
Understanding the anatomy of JavaScript code requires recognizing how statements and expressions work together. For example, a variable declaration statement can include an expression that assigns a value. Loop statements can use expressions to determine the number of iterations. This integration allows for dynamic functionality in JavaScript programming.
Why is it important to understand statements and expressions in JavaScript?
By mastering statements and expressions in JavaScript, you can write code that is not only efficient but also easier to debug and maintain. Each concept is a stepping stone in your journey toward becoming a proficient JavaScript developer.