Reserved Words in JavaScript

JavaScript, one of the most widely-used programming languages, has a structured system of rules to ensure clean and functional code. One key concept developers must understand is reserved words. These words hold special significance in the JavaScript language and cannot be used as identifiers like variable names, function names, or labels.
In this section, we’ll cover the following topics:
- Understanding Reserved Words
- Tips for Avoiding Syntax Errors
What Are Reserved Words?
Reserved words are terms that JavaScript has designated for its internal
operations. For instance, words like return
, if
, and
function
signal specific actions within the code. Using these
words outside their intended context disrupts the flow of execution and
results in errors.
Reserved Words in ECMAScript 2024
ECMAScript 2024, the latest standard for JavaScript, has introduced updates to its list of reserved words. These additions and modifications ensure compatibility with modern programming practices and extend the language’s functionality.
The set of reserved keywords in ECMAScript 2024 includes:
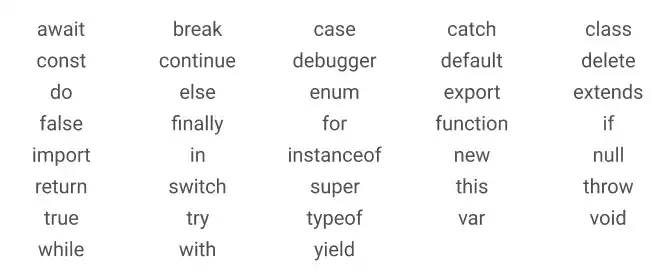
Developers must memorize these to avoid unintentional misuse.
Words Reserved for Future Use
To allow for future growth, JavaScript reserves additional words for potential
features. These include abstract
, boolean
,
byte
, char
, double
, final
,
float
, goto
, int
, long
,
native
, short
, synchronized
,
throws
, transient
, and volatile
. While
not active, using these words can still trigger errors.
Tips for Avoiding Syntax Errors
Avoiding syntax errors caused by reserved words is crucial for smooth coding. By adopting best practices, developers can steer clear of common pitfalls.
Examples of Misuse
A typical misuse might involve naming a variable class
:
// Incorrect
let class = "JavaScript";
// Correct
let className = "JavaScript";
Here, using class
as a variable name leads to an error since it
is reserved for defining classes in JavaScript.
Debugging Reserved Word Conflicts
If you encounter a reserved word conflict, debugging tools can help identify the issue. Tools like Chrome DevTools and Node.js's debugging capabilities provide clear syntax error messages, pointing directly to the problem.
Reference links: