Pseudo Class in CSS

In the world of web development, creating an interactive and dynamic user experience is essential. CSS pseudo classes make this possible by allowing developers to apply styles based on the state or interaction of an element. Whether you want to highlight a button when hovered or apply specific styles to the first child of an element, pseudo classes enable these features without the need for JavaScript. In this guide, we’ll explore how to leverage pseudo classes for better user experience and interactivity.
In this section, we’ll cover the following topics:
- What Are Pseudo Classes in CSS?
- Utilizing Pseudo Classes with AI
- Best Practices for Using Pseudo Classes
What Are Pseudo Classes in CSS?
Pseudo classes in CSS are special selectors that allow you to style elements based on their state, such as when a user hovers over a button or focuses on a form input. They enable you to design elements dynamically based on interactions or specific conditions within the document. This eliminates the need for scripting and helps you manage the user experience directly with CSS.
Commonly Used Pseudo Classes in CSS
Let’s take a closer look at some of the most commonly used pseudo classes and see how they function with real-life examples.
hover – Creating Dynamic Hover Effects
The hover
pseudo class is triggered when the user hovers their cursor over an element. It is often used for creating interactive buttons, links, and images that respond to user interaction.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="code-2.css" />
<title>Hover Effect Example</title>
</head>
<body>
<button class="hover-btn">Hover Over Me</button>
</body>
</html>
button.hover-btn {
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
}
button.hover-btn:hover {
background-color: #0056b3;
}
In this example, the button’s background color changes when the user hovers over it, providing an interactive experience.
Other commonly Used Pseudo Classes in CSS
Here are some of the most commonly used pseudo classes in CSS besides hover
:
:focus
: Applies styling when an element, like an input field, gains focus (e.g., when selected with the mouse or keyboard). This is especially useful for improving accessibility.:active
: Targets an element when it’s being actively clicked or pressed. Often used for button or link styling during the click action.:nth-child(n)
: Selects elements based on their position within a parent element, with n representing the position. As this pseudo class has unique usages as a function, we’ll deep dive into this in the next guide.:first-child
: Selects the first child of a parent element, allowing unique styling for the first item in a list or container.:last-child
: Targets the last child of a parent element, useful for adding unique styling to the final item in a container.:not(selector)
: Selects all elements that do not match a given selector, useful for excluding elements from a styling rule.:checked
: Applies styling to checkboxes or radio buttons when they are selected.:disabled
: Targets elements, such as form inputs, that are disabled, often used to change their appearance to indicate they’re inactive.:visited
: Styles links that the user has already visited, helping differentiate them from unvisited links.
How Pseudo Classes Enhance Web Interactivity
Pseudo classes allow developers to enhance user interaction by providing immediate feedback when a user interacts with elements. For example, when a user hovers over or clicks on a button, the styles can change dynamically, indicating that the element is clickable or active.
Differences Between Pseudo Classes and Pseudo Elements
While pseudo classes target the state of an element (like hover or focus), pseudo elements target specific parts of an element, such as the first line or first letter of a paragraph. For instance, ::before
and ::after
are pseudo elements that allow you to insert content before or after an element, whereas hover
and focus
are pseudo classes used to change styles based on user interaction.
Utilizing Pseudo Classes with AI
In today’s fast-paced development environment, using AI to assist with coding can help streamline the process. AI models can generate sample code for various pseudo classes, saving developers time and effort.
Preparing for Practice Files
This course takes a hands-on approach, allowing you to apply the techniques covered in real-world scenarios. We'll be using a structured folder layout for practice. Before proceeding with the examples, please ensure the following files are prepared:
/your-project-folder/
├── 04-04-pseudo-class-in-css/ (<- sub-folder)
├── example-1.css
├── example-1.html
├── example-2.css
├── example-2.html
├── example-3.css
├── example-3.html
├── example-4.css
├── example-4.html
For your convenience, these files are also available on our GitHub repository. You can download the practice files to follow along with the case studies presented in this guide.
AI Case 1: hover – Creating Dynamic Hover Effects
Let’s see how AI can help generate code for hover effects. Using AI, we can quickly create code for hover states, making it easy to style interactive buttons.
Sample AI prompt:
Generate HTML and CSS code for a button that changes background color when hovered over.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="example-1.css" />
<title>Hover Effect Example</title>
</head>
<body>
<button class="hover-btn">Hover Over Me</button>
</body>
</html>
button.hover-btn {
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
}
button.hover-btn:hover {
background-color: #0056b3;
}
Instructions to see the results:
- Save the code above in
example-1.html
andexample-1.css
in the04-04-pseudo-class-in-css
folder. - Open
example-1.html
in your browser to view the hover effect on the button.
Visit this link to see how it looks in your web browser.
AI Case 2: focus – Enhancing Form Usability and Focused Elements
The focus
pseudo class is useful for enhancing the usability of forms. It is triggered when a user clicks or focuses on an input field.
Sample AI prompt:
Generate HTML and CSS code for a form input field that changes border color when it receives focus.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="example-2.css" />
<title>Focus Effect Example</title>
</head>
<body>
<input type="text" class="focus-input" placeholder="Click to focus" />
</body>
</html>
input.focus-input {
padding: 10px;
border: 2px solid #ccc;
border-radius: 4px;
}
input.focus-input:focus {
border-color: #007bff;
}
Instructions to see the results:
- Save the code in
example-2.html
andexample-2.css
. - Open
example-2.html
in your browser and click on the input field to see the focus effect.
Watch this video to see what it looks like.
Visit this link to see how it looks in your web browser.
AI Case 3: Combining Multiple Pseudo Classes for Complex Styles
Multiple pseudo classes can be combined to apply styles in more specific scenarios. For example, you can style an element when it is both hovered and focused.
Sample AI prompt:
Generate HTML and CSS code that styles a button when it is both hovered and focused.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="example-3.css" />
<title>Combined Pseudo Classes Example</title>
</head>
<body>
<button class="combined-btn">Hover and Focus Me</button>
</body>
</html>
button.combined-btn {
padding: 10px 20px;
background-color: #28a745;
color: white;
border: none;
border-radius: 5px;
}
button.combined-btn:hover,
button.combined-btn:focus {
background-color: #218838;
}
Instructions to see the results:
- Save the code in
example-3.html
andexample-3.css
. - Open
example-3.html
in your browser and interact with the button by hovering and focusing to see the combined effect.
Visit this link to see how it looks in your web browser.
AI Case 4: Using not for Conditional Styling
The not
pseudo class allows you to apply styles to all elements except those specified in the condition.
Sample AI prompt:
Generate HTML and CSS code that styles all buttons except one with a specific class.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="example-4.css" />
<title>Not Pseudo Class Example</title>
</head>
<body>
<button class="btn">Button 1</button>
<button class="btn">Button 2</button>
<button class="btn no-style">Button 3</button>
</body>
</html>
button.btn:not(.no-style) {
background-color: #ffc107;
color: white;
border: none;
padding: 10px 20px;
cursor: pointer;
}
Instructions to see the results:
- Save the code in
example-4.html
andexample-4.css
. - Open
example-4.html
in your browser to see how the third button is styled differently.
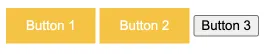
Visit this link to see how it looks in your web browser.
Best Practices for Using Pseudo Classes in CSS
Using pseudo classes effectively can significantly enhance the interactivity and usability of your website. Here are some best practices to follow when applying pseudo classes in CSS.
- Use Specificity Wisely: Limit the use of pseudo classes to elements that truly benefit from dynamic styling. This avoids overcomplicating the CSS and keeps your stylesheets maintainable.
- Combine Pseudo Classes for Complex Effects: Combining pseudo classes like
:hover
and:focus
can create more nuanced interactions. For example, styling a button differently when it is both hovered and focused enhances accessibility. - Prioritize Accessibility: Pseudo classes like
:focus
improve accessibility by clearly indicating which form elements are active, especially for keyboard users. Ensure these styles are highly visible. - Keep Styling Consistent Across States: When using pseudo classes such as
:hover
and:active
, maintain a logical flow in color and design transitions, so users experience a smooth interaction with each element. - Use Pseudo Classes Instead of JavaScript Where Possible: Pseudo classes can handle many dynamic styling needs without JavaScript, reducing load times and simplifying code.
- Test Across Devices: Different devices and browsers may handle pseudo classes differently. Test your styles on various screens to ensure a consistent and reliable user experience.
Applying these practices will help create more engaging and accessible user interfaces. Remember, each carefully chosen pseudo class contributes to an intuitive, responsive design.