nth-child

The nth-child()
pseudo-class in CSS is a powerful selector that enables developers to target specific elements within a parent container based on their position. It can be used for a variety of design purposes, such as alternating row colors in tables, creating responsive grid layouts, or applying unique styles to certain elements without adding additional classes. By mastering the nth-child()
selector, you can streamline your CSS and build more efficient, dynamic layouts.
In this guide, we’ll cover how to use the nth-child()
pseudo-class in CSS, explore practical use cases, and provide hands-on examples, including AI-generated code to enhance your workflow.
In this section, we’ll cover the following topics:
- What is nth-child() in CSS?
- Utilizing nth-child() Pseudo Classes with AI
- Best Practices for Using nth-child() Pseudo Classes
What is nth-child() in CSS?
The nth-child()
pseudo-class allows developers to select elements based on their order within their parent element. It’s a flexible and dynamic tool that can target any HTML element, from list items to table rows, enabling precise control over styling.
Basic Syntax of nth-child() in CSS
The basic syntax for using nth-child()
is as follows:
Example:
element:nth-child(n) {
/* Styles */
}
element
represents the HTML tag to be targeted (such asli
,tr
, ordiv
).n
refers to the position of the child element within the parent container.
You can use several types of values for n
:
- Numeric values: Selects a specific child element, like
nth-child(2)
for the second child. - Keywords:
nth-child(odd)
selects all odd elements, andnth-child(even)
selects all even elements. - Formulas: Using
2n
, you can target every second element, while3n+1
would select every third element starting with the first.
nth-child() CSS Use Cases
nth-child()
is highly versatile and can be used in various design scenarios. Below are some common use cases.
Example: Styling Alternate Rows in a Table
One of the most common uses for nth-child()
is styling alternating table rows.
Example:
<table>
<tr>
<td>Row 1</td>
</tr>
<tr>
<td>Row 2</td>
</tr>
<tr>
<td>Row 3</td>
</tr>
<tr>
<td>Row 4</td>
</tr>
</table>
<style>
tr:nth-child(even) {
background-color: #8ddbff;
}
</style>
In this example, nth-child(even)
is used to style every even table row, creating a striped effect.
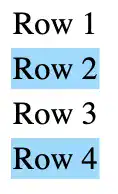
Example: Targeting Every Third Item
To target every third list item, you can use the formula 3n
.
Example:
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
<li>Item 6</li>
</ul>
<style>
li:nth-child(3n) {
color: red;
}
</style>
This code turns every third list item red, allowing for selective styling without the need for extra classes or IDs.
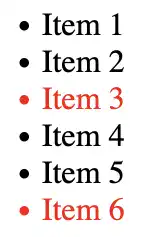
nth-child() vs. nth-of-type(): Key Differences
While nth-child()
targets elements based on their position within a parent, nth-of-type()
selects elements based on their type. This distinction is important when working with mixed content types.
Example:
p:nth-child(2) {
color: blue;
}
p:nth-of-type(2) {
color: red;
}
If the second child of a parent is not a <p>
element, nth-child(2)
will not select it, whereas nth-of-type(2)
specifically targets the second <p>
element regardless of its position.
For more details, refer to the case studies below.
Utilizing nth-child() Pseudo Classes with AI
Leveraging AI for coding can speed up your development process by generating efficient and functional CSS code. In this section, we’ll walk through examples of how AI can help you use the nth-child()
pseudo-class in CSS.
Preparing for Practice Files
This course takes a hands-on approach, allowing you to apply the techniques covered in real-world scenarios. We'll be using a structured folder layout. Before proceeding with the examples, please ensure the following files are prepared:
/your-project-folder/
├── 04-05-nth-child/ (<- sub-folder)
├── example-1.css
├── example-1.html
├── example-2.css
├── example-2.html
├── example-3.css
├── example-3.html
├── example-4.css
├── example-4.html
For your convenience, these files are also available on our GitHub repository. You can download the practice files to follow along with the case studies presented in this guide.
AI Case 1: Styling Alternate Rows with nth-child()
In this case study, we’ll use nth-child()
to style alternate rows of a table.
Sample AI prompt:
Generate CSS code to style every even row in a table with a light blue background.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Alternate Row Styling</title>
<link rel="stylesheet" href="example-1.css" />
</head>
<body>
<table>
<tr>
<td>Row 1</td>
</tr>
<tr>
<td>Row 2</td>
</tr>
<tr>
<td>Row 3</td>
</tr>
<tr>
<td>Row 4</td>
</tr>
</table>
</body>
</html>
tr:nth-child(even) {
background-color: lightblue;
}
Instructions to see the results:
- Save the code above in
example-1.html
andexample-1.css
in the04-05-nth-child
folder. - Open
example-1.html
in your browser to view the table with alternating row colors.
Visit this link to see how it looks in your web browser.
AI Case 2: Targeting Specific Elements with nth-child()
In this case, we’ll target specific elements in a list using nth-child()
.
Sample AI prompt:
Generate CSS code to style the 2nd and 4th list items with a background color of #ffcccc.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Target Specific List Items</title>
<link rel="stylesheet" href="example-2.css" />
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
</body>
</html>
li:nth-child(2),
li:nth-child(4) {
background-color: #ffcccc;
}
Instructions to see the results:
- Save the code above in
example-2.html
andexample-2.css
in the04-05-nth-child
folder. - Open
example-2.html
in your browser to view the styled list.
Visit this link to see how it looks in your web browser.
AI Case 3: Using nth-child() with Class Selectors
Next, we’ll explore how to use nth-child()
alongside class selectors.
Sample AI prompt:
Generate CSS code to style the 3rd paragraph within a container that has the class text-container.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Using Class Selectors</title>
<link rel="stylesheet" href="example-3.css" />
</head>
<body>
<div class="text-container">
<p>Paragraph 1</p>
<p>Paragraph 2</p>
<p>Paragraph 3</p>
</div>
</body>
</html>
.text-container p:nth-child(3) {
font-weight: bold;
}
Instructions to see the results:
- Save the code above in
example-3.html
andexample-3.css
in the04-05-nth-child
folder. - Open
example-3.html
in your browser to see the third paragraph styled with bold text.
Visit this link to see how it looks in your web browser.
AI Case 4: Understanding the Difference Between nth-child() and nth-of-type()
In this case study, we’ll explore how nth-child()
and nth-of-type()
behave differently when targeting elements within a parent container.
Sample AI prompt:
Generate an HTML and CSS code example to demonstrate the difference between nth-child() and nth-of-type() by targeting list items with mixed content (paragraphs and lists).
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>nth-child() vs nth-of-type()</title>
<link rel="stylesheet" href="example-4.css" />
</head>
<body>
<div class="container">
<p>Paragraph 1</p>
<p>Paragraph 2</p>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<p>Paragraph 3</p>
<ul>
<li>Item 4</li>
<li>Item 5</li>
</ul>
<p>Paragraph 4</p>
</div>
</body>
</html>
/* Using nth-child to target the second child, which could be any element type */
.container p:nth-child(2) {
color: red;
}
/* Using nth-of-type to target the second paragraph element specifically */
.container p:nth-of-type(2) {
background-color: yellow;
}
/* Using nth-child to target the third child, regardless of type */
.container ul li:nth-child(3) {
font-weight: bold;
}
/* Using nth-of-type to target the third list item specifically within each list */
.container ul li:nth-of-type(2) {
color: blue;
}
Explanation:
- The
nth-child(2)
rule targets the second child in the container, which happens to be a paragraph, and changes its text color to red. - The
nth-of-type(2)
rule targets the second paragraph element regardless of its position in the container, highlighting it with a yellow background. - For the lists,
nth-child(3)
targets the third child of the parent (in this case, a list item), which makes the third list item bold. - The
nth-of-type(2)
rule targets the second list item in each list, turning the text blue.
Instructions to see the results:
- Save the code above in
example-4.html
andexample-4.css
in the04-05-nth-child
folder. - Open
example-4.html
in your browser to view hownth-child()
andnth-of-type()
behave differently with mixed elements.
Visit this link to see how it looks in your web browser.
Best Practices for Using nth-child() Pseudo Classes
Mastering the nth-child()
pseudo-class can significantly improve the efficiency and readability of your CSS, especially for dynamic layouts and complex styling needs. Here are some best practices to keep in mind:
- Optimize Selector Specificity: Use
nth-child()
to target elements without extra classes or IDs. This keeps your HTML cleaner and allows CSS to handle element selection effectively. - Choose Meaningful Patterns: Utilize formulas like
nth-child(odd)
,nth-child(even)
, ornth-child(3n+1)
to apply consistent styles across specific patterns in lists, tables, or grids. This enhances visual consistency without repetitive code. - Combine with Other Selectors: For refined targeting, pair
nth-child()
with classes or other pseudo-classes. For instance,.text-container p:nth-child(3)
targets only the third paragraph inside a specified container. - Consider Accessibility: Ensure visual patterns created with
nth-child()
support readability and accessibility, especially in alternating row colors or other styles that affect layout clarity.
By following these best practices, you’ll harness the full potential of nth-child()
for flexible and maintainable CSS layouts.