CSS Variable: Creating CSS Custom Properties

CSS variables, also known as custom properties, offer a powerful way to enhance your CSS code by making it more dynamic and maintainable. These variables allow you to store values like colors, fonts, and spacing once and reuse them throughout your stylesheet. By implementing CSS variables, developers can easily manage styles, make updates efficiently, and create consistent themes across an entire website or application. This guide will take you through the essentials of CSS variables, including how to define and use them, how they can be enhanced using AI, and best practices to get the most out of custom properties.
In this section, we’ll cover the following topics:
- What Are CSS Variables?
- How to Create and Use CSS Variables
- Creating and Using CSS Variables with AI
- Best Practices for CSS Variable
What Are CSS Variables?
CSS variables, or custom
properties, are a way to assign values to a property once and reuse that value
across your stylesheet. This can significantly reduce redundancy in your CSS
and make it easier to maintain a consistent design. Custom properties are
declared with a --
prefix and can store any valid CSS
value, such as colors, lengths, or font sizes.
Understanding CSS Custom Properties
CSS custom properties function
much like variables in programming languages. You declare them in your
stylesheet and then reference them wherever needed using the var()
function. This makes managing large stylesheets easier and allows for flexible
design updates. For example, you can create a custom property for a primary
color and update it across your entire site by changing one line of code.
Benefits of Using CSS Variables
The key advantage of CSS variables is their ability to simplify and streamline your stylesheets. By using variables, you avoid the need to manually update multiple instances of the same value. Additionally, CSS variables make it easier to implement theming and respond to design changes efficiently. Custom properties also support inheritance, meaning they can be used and redefined within child elements for more complex designs.
Basic Syntax of CSS Variables
CSS variables are defined
within the :root
or any specific element in your stylesheet, making them globally or
locally accessible. Here’s the basic syntax:
:root {
--main-color: #3498db;
--font-size: 16px;
}
body {
color: var(--main-color);
font-size: var(--font-size);
}
This example defines two CSS
variables, --main-color
and --font-size
, which are applied to the body
element. The var()
function is used to reference the variables within your styles.
Browser Support and Best Practices for CSS Variables
CSS variables are supported by all modern browsers, including Chrome, Firefox, and Edge. However, older browsers like Internet Explorer do not support them, so it’s important to include fallback values when necessary. You can ensure compatibility by using traditional CSS properties alongside custom properties. For example:
h1 {
color: #000; /* fallback for older browsers */
color: var(--main-color);
}
This way, older browsers will still display the fallback color even if they don’t support the CSS variable.
How to Create and Use CSS Variables
To effectively use CSS variables, you need to understand how to define them globally or locally, as well as how to update them for different selectors. This section will guide you through the process of creating and applying variables across your stylesheets.
Defining CSS Custom Properties
CSS custom properties are
usually defined in the :root
selector, making them available
globally across the entire document. For example:
:root {
--primary-color: #ff5733;
--secondary-color: #4caf50;
}
By declaring variables in the :root
,
you can use these properties anywhere in your stylesheet.
Using CSS Variables in Your Stylesheets
Once a variable is defined, it
can be referenced in any CSS rule using the var()
function. This makes it easy to apply the same value to multiple selectors
without repeating the value.
button {
background-color: var(--primary-color);
}
p {
color: var(--secondary-color);
}
Local vs Global CSS Variables
While global variables are
defined in the :root
and are accessible throughout your
entire document, you can also define variables locally within a specific
selector. Local variables will only be available within the scope of that
selector, offering greater control for component-based designs.
.card {
--card-padding: 20px;
padding: var(--card-padding);
}
Updating CSS Custom Properties in Different Selectors
CSS variables can be updated and overridden within different selectors. This feature allows you to customize specific components, such as buttons or headings, while still maintaining a global theme.
:root {
--main-color: #3498db;
}
h1 {
color: var(--main-color);
}
button {
--main-color: #e74c3c; /* override the global variable */
background-color: var(--main-color);
}
In this example, the --main-color
is set globally, but the button element overrides it with a different
color.
Creating and Using CSS Variables with AI
AI tools can significantly enhance the process of creating and managing CSS variables by automating repetitive tasks and generating consistent designs. In this section, we’ll explore how AI can assist in defining and applying CSS variables for theming, spacing, and more.
Preparing for Practice Files
This course takes a hands-on approach, allowing you to apply the techniques covered in real-world scenarios. We'll be using a structured folder layout. Before proceeding with the examples, please ensure the following files are prepared:
/your-project-folder/
├── 07-01-css-variable/ (<- sub-folder)
├── example-1.css
├── example-1.html
├── example-2.css
├── example-2.html
For your convenience, these files are also available on our GitHub repository. You can download the practice files to follow along with the case studies presented in this guide.
AI Case 1: Theming and Color Management
Managing theme colors across a website or application becomes much simpler with CSS variables. This case focuses on creating custom properties for primary, secondary, and accent colors, which can be reused throughout the design to maintain consistent theming.
Sample AI prompt:
Generate a CSS file with custom properties for theming, including primary, secondary, and accent colors. Use the :root selector to define the variables with a well-balanced color palette.
Sample code output:
<html>
<head>
<link rel="stylesheet" href="example-1.css" />
</head>
<body>
<header>
<h1>Website Theme Example</h1>
<p>
This is an example of applying custom theme colors using CSS variables.
</p>
</header>
<button>Click Me</button>
</body>
</html>
:root {
--primary-color: #2c3e50; /* Dark Slate Blue */
--secondary-color: #18bc9c; /* Light Turquoise */
--accent-color: #f39c12; /* Bright Amber */
}
body {
background-color: var(--primary-color);
color: var(--secondary-color);
font-family: Arial, sans-serif;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
header {
text-align: center;
padding: 50px;
}
h1 {
color: var(--accent-color);
}
.button-container {
display: flex;
justify-content: center;
}
button {
background-color: var(--secondary-color);
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
font-size: 1.2rem;
}
button:hover {
background-color: var(--accent-color);
cursor: pointer;
}
Instructions to see the results:
- Save the code above in
example-1.html
andexample-1.css
in the07-01-css-variable
folder. - Open
example-1.html
in your browser to see how the theme colors are applied.
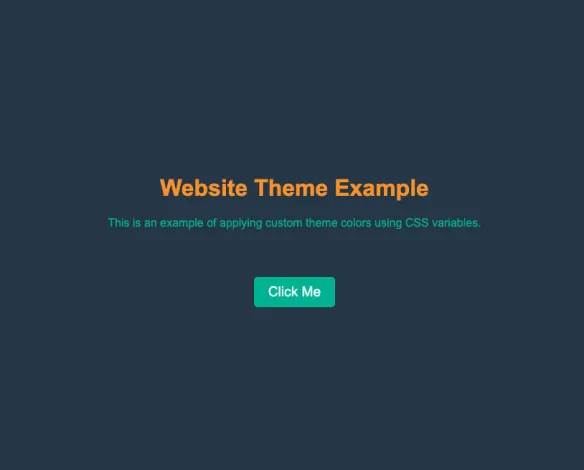
Visit this link to see how it looks in your web browser:
How to Test and Experiment with Color Variables
- Change the Color Values: To understand how CSS variables work, try changing the color
values in
example-1.css
. For example, update the--primary-color
from#2C3E50
(Dark Slate Blue) to#1ABC9C
(Turquoise) and the--accent-color
from#F39C12
(Bright Amber) to#E74C3C
(Bright Red). Save the changes and reload the page to see how the theme changes instantly across the entire layout. - Observe the Changes: After updating the variables, observe how the background color (primary color) changes to turquoise and how the heading and button styles (accent color) change to red. These modifications give the webpage a new look with just a few updates to the CSS variables.
AI Case 2: Reusable Spacing and Sizing
CSS variables can be particularly useful for managing spacing and sizing across different elements. This case demonstrates how to create reusable variables for margins, padding, and container sizes to maintain consistent layouts throughout a project. In this example, you’ll learn how to apply and test spacing variables and see how they respond to window resizing.
Sample AI prompt:
Generate CSS variables for reusable spacing, including margin, padding, and size control. Apply these variables to elements for consistent layout spacing.
Sample code output:
<html>
<head>
<link rel="stylesheet" href="example-2.css" />
</head>
<body>
<div class="container">
<h1>Reusable Spacing Example</h1>
<p>
This example demonstrates consistent margins and padding using CSS
variables.
</p>
<button>Click Me</button>
</div>
</body>
</html>
:root {
--default-margin: 20px;
--default-padding: 15px;
--button-padding: 10px 20px;
--container-width: 80%;
}
body {
margin: var(--default-margin);
padding: var(--default-padding);
}
.container {
width: var(--container-width);
margin: auto;
padding: var(--default-padding);
}
button {
padding: var(--button-padding);
border: none;
background-color: #3498db;
color: white;
border-radius: 5px;
}
Instructions to see the results:
- Save the code above in
example-2.html
andexample-2.css
in the07-01-css-variable
folder. - Open
example-2.html
in your browser to see how spacing and sizing are applied consistently across elements.
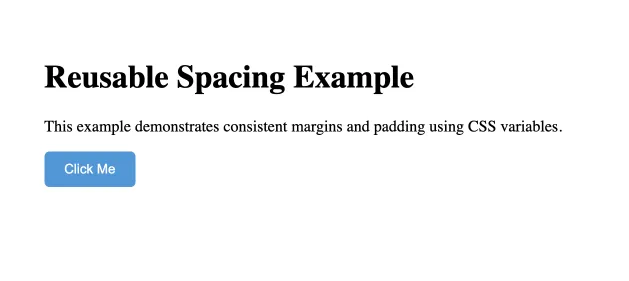
Visit this link to see how it looks in your web browser:
How to Test and Experiment with Variables
- Resize your browser window: You can test
how the spacing variables behave by shrinking or expanding the window
size. The container’s width is defined by the
--container-width
variable, and as you resize the window, you will notice that the content adjusts dynamically within the defined container width. - Experiment with variable values: To fully
understand how CSS variables work, try changing the values of the spacing
variables in
example-2.css
. For example, update--default-margin
from20px
to40px
or--container-width
from80%
to60%
. Save the changes and reload the page in your browser to see how the layout adjusts. - Observe the changes: After updating the variables, observe how the layout changes. The larger margin will create more space around the body of the page, while reducing the container width will make the content area narrower.
- Test button padding: You can also
experiment with the
--button-padding
variable to change the padding around the button. Try increasing the padding to20px
40px
to see how it affects the button size.
These
steps will help you see the effect of using CSS variables and understand how
modifying a single value can influence the entire layout. By adjusting
variables like --default-margin
, --default-padding
, and --container-width
,
you can maintain consistency in your design while testing responsiveness and
layout behavior.
Best Practices for CSS Variables
CSS variables, or custom properties, can transform the way you manage styles in your stylesheet, enabling flexibility and ease of updates. Follow these best practices to get the most out of CSS variables and create maintainable, responsive designs.
-
Define Variables Globally: Use the
:root
selector to declare variables that will be used throughout your stylesheet. This centralizes values, making it easy to manage global themes and styles. -
Use Consistent Naming Conventions: Name variables with clear, descriptive prefixes
like
--color-
,--font-
, or--size-
to indicate their purpose, aiding readability and team collaboration. - Leverage Local Overrides When Needed: While global variables are useful for consistent theming, define local variables within specific components when you need isolated adjustments without affecting the entire document.
- Add Fallbacks for Compatibility: Since CSS variables aren't supported by all older browsers, provide fallback values for essential styles to ensure broader compatibility.
- Optimize for Theming and Customization: Use CSS variables to create customizable themes. Define core color and font variables in one place, allowing you to easily adjust the appearance of your entire site by changing a few values.
- Use Variables for Reusable Spacing and Sizing: Define variables for margins, padding, and dimensions to maintain consistency across layouts and ensure responsive design adjustments are easy to apply.
These practices will help you create adaptable and maintainable stylesheets, making CSS variables a powerful tool in your web development toolkit.