Chapter 7. Manipulating Web Pages with JavaScript

JavaScript is the cornerstone of dynamic web experiences, allowing developers to make web pages interactive, responsive, and engaging. In this chapter, we delve into the key techniques and concepts that empower you to manipulate web pages effectively using JavaScript. From understanding the Browser Object Model (BOM) and Document Object Model (DOM) to mastering advanced event handling techniques, this guide equips you with the skills to enhance user interactions on any device, whether desktop or mobile.
By exploring real-world applications, best practices, and a wide variety of events—mouse, keyboard, touch, and even custom events—you’ll gain a comprehensive understanding of how JavaScript transforms static pages into dynamic applications. Whether you're building forms, implementing animations, or enhancing multimedia elements, this chapter has everything you need to get started.
Web Page Manipulation with JavaScript
JavaScript enables the creation of dynamic and responsive web pages by manipulating HTML, CSS, and browser elements. This section provides an accessible overview of its core capabilities, emphasizing its pivotal role in transforming static content into interactive web experiences. The focus here is on its practical applications in web development, specifically in building engaging and user-friendly interfaces.
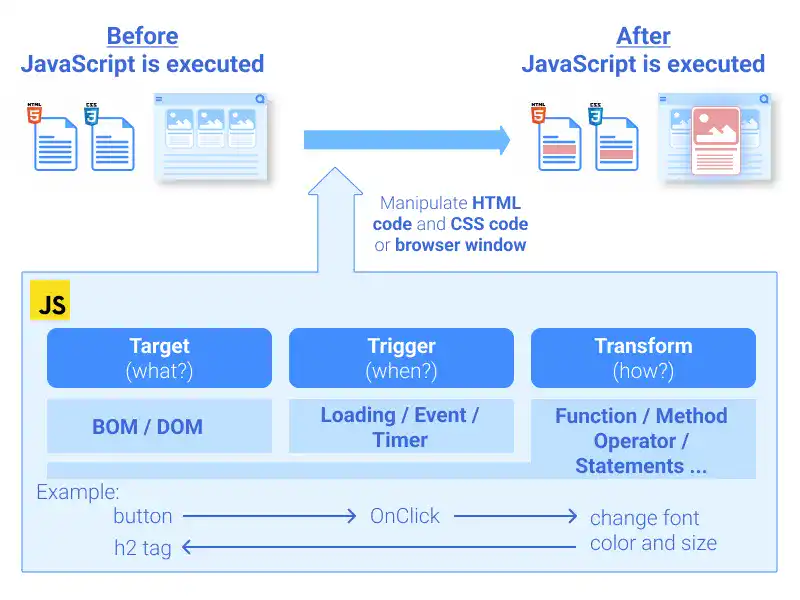
JavaScript's Role: Transforming Static into Dynamic
The first key concept is understanding that JavaScript manipulates HTML, CSS, and browser windows to enable dynamic changes to otherwise static web pages. When a browser renders a page, it processes JavaScript to modify HTML elements, apply new CSS styles, or control browser components like pop-up windows. This processing transforms the user experience, making web pages interactive and visually engaging.
The “Where, When, and How” of JavaScript
A practical way to understand JavaScript is by breaking down its event cycle into three aspects: where, when, and how. This framework provides a structured approach to grasping how JavaScript operates:
Where? (Target)
JavaScript interacts with specific parts of a webpage or browser. These targets are defined using two critical models:
- Browser Object Model (BOM): Enables interaction with browser-specific elements like the window or navigation history.
- Document Object Model (DOM): Represents the HTML and CSS structure, allowing JavaScript to manipulate elements like headings, buttons, and forms.
When? (Trigger)
JavaScript actions are triggered under three primary circumstances:
- Page Load: Automatically when the browser processes JavaScript on a webpage.
- User Actions: Events like clicking a button or hovering over a link are defined using event handlers or listeners.
- Timers: Actions based on predefined intervals or delays, such as animations or countdowns.
How? (Execution)
Finally, JavaScript defines the logic and actions that drive changes. Whether updating HTML content, applying CSS styles, or initiating browser actions, this “how” aspect involves writing functions, using methods, and implementing statements. These logical constructs form the backbone of JavaScript's ability to bring web pages to life.
By focusing on where, when, and how, developers can demystify JavaScript and leverage its full potential to build dynamic, user-friendly websites.
What We Cover in This Chapter
In this chapter, we’ll explore the essential techniques for manipulating web pages with JavaScript, focusing on the DOM, BOM, and event handling. By the end, you’ll have a strong understanding of how to use JavaScript to create interactive, responsive, and user-friendly web applications. The following topics are covered:
BOM (Browser Object Model) and DOM (Document Object Model)
The Browser Object Model (BOM) and Document Object Model (DOM) are essential tools for web developers. The BOM focuses on browser-specific tasks like managing windows and history, while the DOM enables interaction and modification of HTML and CSS elements. Together, they form the foundation for building dynamic and interactive websites.
getElementBy*() vs. querySelector() – Choosing the Right Method
This section contrasts getElementBy*()
methods with
querySelector()
and querySelectorAll()
. It explains
their differences in syntax, performance, and functionality, helping
developers make informed decisions when selecting elements in the DOM.
Event Handlers and Event Listeners in JavaScript
Explore the differences between event handlers and event listeners, their use cases, and how to implement them effectively. This section includes examples of advanced techniques like event delegation and managing propagation.
JavaScript Event Object
The JavaScript Event Object provides crucial information about user
interactions, such as event type and target element. This section details how
to use its properties and methods, including preventDefault()
and
stopPropagation()
, to control behavior and build responsive
features.
Mouse Events
Mouse events enable dynamic interactions like clicking, dragging, and
hovering. This section covers common events such as click
,
mouseover
, and contextmenu
, and explains how to
implement and optimize them for seamless user experiences.
Keyboard Events
Keyboard events allow developers to capture and respond to key presses for
features like shortcuts, navigation, and input validation. This section
discusses key properties like event.key
and best practices for
accessibility and performance.
Focus and Blur Events
Focus and blur events help manage user interactions with form elements and buttons. Learn how to highlight inputs, show tooltips, or prevent accidental actions using these events for an improved user experience.
Form Events
Form events like submit
and change
enable developers
to handle user input dynamically. This section covers validation techniques,
error handling, and enhancing forms to create user-friendly interfaces.
Window Events
Window events like resize
and scroll
allow
developers to respond to browser-level actions. This section explains how to
leverage these events to create responsive layouts and improve app usability.
Touch Events (For Mobile Devices)
Touch events are crucial for building mobile-friendly applications. This
section introduces events like touchstart
and
touchmove
and demonstrates their use for gestures and
interactions on touch devices.
Drag and Drop Events
Drag-and-drop functionality enhances user interactivity. This section outlines how to implement drag-and-drop using JavaScript events, covering both basic and advanced use cases.
Animation Events
Animation events like animationstart
and
animationend
are essential for managing CSS animations
dynamically. Learn how to synchronize animations with user interactions for
engaging visual effects.
Media, Network, and Advanced JavaScript Events
This section introduces events related to media playback, network activity, and advanced JavaScript use cases. Topics include handling video playback events and monitoring resource loading for performance optimization.
JavaScript Custom Events
Custom events enable developers to define and trigger unique actions. This section explores creating and dispatching custom events to build highly interactive and modular applications.
Learn offline for better focus!
A book for this course is available on Amazon.
Learn JavaScript Coding with AI
Revolutionize Your Learning with ChatGPT in This Beginner's JavaScript Book
Get the Book Now