Case Style for JavaScript – Camel Case

Case styles play a significant role in ensuring clarity and consistency in coding. One of the most widely adopted styles in JavaScript is Camel Case, known for its simplicity and ease of use. This guide will explore case styles, especially Camel Case, and explain why it’s important for effective JavaScript coding.
In this section, we’ll cover the following topics.
- What is Case Style?
- How to Use Camel Case in JavaScript
What is Case Style?
Case style refers to the way words are written in programming to make code more readable and easier to maintain. By structuring variables, functions, and other elements systematically, developers can follow and debug code more effectively.
Why Does Case Style Matter?
Case style isn’t just about aesthetics—it’s about functionality. Consistent use of case styles:
- Enhances readability and collaboration.
- Prevents bugs caused by mistyped variable names.
- Aligns with industry standards, helping developers transition between projects seamlessly.
Commonly Used Case Styles
There are several case styles, each suited to specific programming languages and scenarios.
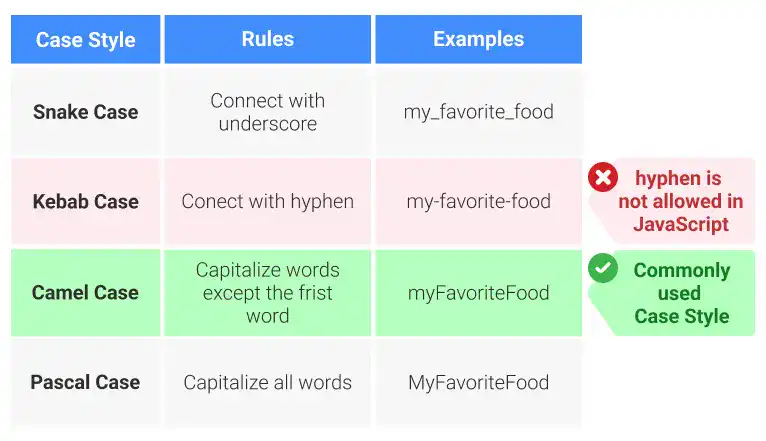
Let’s break them down:
Snake Case
In Snake Case, words are separated by underscores (_
) to create
clear and readable identifiers. This style is particularly useful for
scenarios where spaces or hyphens are not allowed.
-
Example:
user_name
,access_token
-
Commonly used in: Python, Ruby, and for environment variables (e.g.,
DATABASE_URL
,API_KEY
)
Note: While Snake Case is standard in some languages, it is uncommon in JavaScript for variable or function names. However, it is often used in configuration files, constants, and environment variables due to its compatibility across systems.
Kebab Case
Words are separated by hyphens (-
).
- Example:
user-name
- Commonly used in: HTML, CSS
Note: JavaScript doesn’t support hyphens in identifiers (variable or function names) because - is interpreted as a subtraction operator.
Camel Case
Words are written together, with each word starting with an uppercase letter except the first.
- Example:
userName
- Commonly used in: JavaScript, Java
JavaScript-Specific Usage: Camel case is the standard for naming variables, functions, and object properties.
Pascal Case
Similar to Camel Case, but the first word also starts with an uppercase letter.
- Example:
UserName
- Commonly used in: C#, .NET
JavaScript-Specific Usage: Often used for naming classes and constructors, such as UserProfile or AppConfig.
How to Use Camel Case in JavaScript
Using Camel Case effectively is crucial for writing professional and maintainable JavaScript code. It reduces ambiguity, aligns with community best practices, and ensures compatibility when collaborating with other developers. The rules for writing in Camel Case vary based on the length of the identifier:
Examples with Single Words
When using a single word, Camel Case doesn’t change the capitalization since there’s only one word. Here are some simple examples:
age
(e.g., for storing a user’s age)name
(e.g., for holding a person’s name)
Examples with Two Words
For identifiers with two words, the first word remains lowercase, and the second word starts with an uppercase letter. Examples:
firstName
(e.g., to represent the first name of a user)lastName
(e.g., to represent the last name of a user)
This structure keeps the identifier concise while clearly separating the words visually.
Examples with More Than Two Words
When more than two words are needed, the rule remains the same: capitalize the first letter of each word after the first. This approach ensures readability even for longer identifiers. Examples:
-
totalAnnualRevenue
(e.g., for storing a company’s total revenue over a year) -
isAccountActive
(e.g., for checking whether a user’s account is active)
By following these conventions, you create code that’s intuitive, less prone to errors, and easy for others to understand. Camel Case is a foundational element of clean JavaScript coding, and mastering it will enhance your overall programming skills.
Reference links:
FAQ: Case Style for JavaScript – Camel Case
What is Case Style?
Case style refers to the way words are written in programming to make code more readable and easier to maintain. By structuring variables, functions, and other elements systematically, developers can follow and debug code more effectively.
Why Does Case Style Matter?
Case style isn’t just about aesthetics—it’s about functionality. Consistent use of case styles enhances readability and collaboration, prevents bugs caused by mistyped variable names, and aligns with industry standards, helping developers transition between projects seamlessly.
What are Commonly Used Case Styles?
There are several case styles, each suited to specific programming languages and scenarios. Common styles include Snake Case, Kebab Case, Camel Case, and Pascal Case. Each has its own use cases and is preferred in different programming environments.
How is Camel Case Used in JavaScript?
Camel Case is the standard for naming variables, functions, and object properties in JavaScript. It involves writing words together, with each word starting with an uppercase letter except the first. This style reduces ambiguity and aligns with community best practices.
What are Examples of Camel Case in JavaScript?
Examples of Camel Case include single words like "age" and "name," two-word identifiers like "firstName" and "lastName," and longer identifiers like "totalAnnualRevenue" and "isAccountActive." This structure keeps identifiers concise and visually clear.