Attribute Selector in CSS

CSS attribute selectors offer a versatile way to target and style elements based on their attributes. This allows for more dynamic, flexible design without the need to add classes or IDs to your HTML. By leveraging attribute selectors, you can make your code more efficient and your styling more adaptable to changes in content.
In this guide, we will explore the different types of CSS attribute selectors, their use cases, and best practices. We will also look at how to combine them with other selectors and how AI tools can assist in generating CSS for real-world applications. This comprehensive approach will help you master attribute selectors and apply them effectively in your projects.
In this section, we’ll cover the following topics:
- What is an Attribute Selector in CSS?
- Common Types of Attribute Selectors
- Best Practices for Using Attribute Selectors
- Combining Attribute Selectors with Other CSS Selectors
- Utilizing CSS Attribute Selectors with AI
What is an Attribute Selector in CSS?
An attribute selector is a type of CSS selector that allows you to target elements based on the attributes assigned to them. This means you can style elements not only based on their tags, classes, or IDs but also based on specific attributes or attribute values. For example, you can use an attribute selector to style all links with a particular href
value or all input fields of a certain type
.
Attribute selectors are particularly useful for handling dynamic content, form elements, or any situation where you want to apply styles to elements that share similar attributes. By using them, you can reduce the need for extra classes and make your HTML cleaner.
Common Types of Attribute Selectors
CSS provides several variations of attribute selectors, each designed for different use cases. Here are the most common types:
Basic Attribute Selector:
Targets elements with a specific attribute.
Example:
[disabled] {
background-color: grey;
}
In this example, all elements with the disabled
attribute will have a grey background.
Attribute Selector with Specific Value:
Targets elements where an attribute has a specific value.
Example:
input[type="text"] {
border: 1px solid blue;
}
This selector styles all input
elements with a type
of text
by applying a blue border.
Attribute Selector with Partial Match:
These selectors allow you to target elements whose attribute values partially match the provided value.
Example:
a[href^="https"] {
color: green;
}
The ^=
selector targets all a
(anchor) elements whose href
attribute starts with "https", applying green text color to those links.
Combining Attribute Selectors with Other CSS Selectors
Attribute selectors can be combined with other CSS selectors like class selectors and pseudo-classes for more precise control. This enables you to style elements in a more refined way based on multiple conditions.
Attribute Selectors with Class Selectors
Combining attribute selectors with class selectors allows you to apply styles only to elements that have both a specific class and attribute.
Example:
button.submit[type="submit"] {
background-color: blue;
color: white;
}
In this case, only buttons with the class submit
and a type
attribute set to submit
will be styled with a blue background and white text. This is useful for adding more targeted styles without adding extra markup.
Attribute Selectors with Pseudo-Classes
You can also combine attribute selectors with pseudo-classes like :hover
to apply styles based on user interactions.
Example:
a[href^="https"]:hover {
text-decoration: underline;
}
Here, links whose href
attribute starts with "https" will have an underline applied when the user hovers over them. This is a practical way to improve the user experience for external links.
Utilizing CSS Attribute Selectors with AI
In modern web development, AI tools can assist you in writing CSS attribute selectors more efficiently. With AI code generators like ChatGPT, you can automate the process of writing and testing CSS code, saving time and reducing errors.
Preparing for Practice Files
This course takes a hands-on approach, allowing you to apply the techniques covered in real-world scenarios. We'll be using a structured folder layout. Before proceeding with the examples, please ensure the following files are prepared:
/your-project-folder/
├── 04-02-attribute-selector-in-css/ (<- sub-folder)
├── example-1.css
├── example-1.html
├── example-2.css
├── example-2.html
For your convenience, these files are also available on our GitHub repository. You can download the practice files to follow along with the case studies presented in this guide.
AI Case 1: Styling Input Fields with Attribute Selectors
This case study demonstrates the attribute selector with specific values.
Sample AI prompt:
Generate CSS code to style input fields with the required
attribute, applying a red border.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Attribute Selector Example 1</title>
<link rel="stylesheet" href="example-1.css" />
</head>
<body>
<h2>Form Fields</h2>
<input type="text" required placeholder="Required field" />
<input type="text" placeholder="Optional field" />
</body>
</html>
input[required] {
border: 2px solid red;
}
Instructions to see the results:
- Save the code above in
example-1.html
andexample-1.css
. - Open
example-1.html
in your browser to view the required input field with a red border, while the optional field remains unstyled.
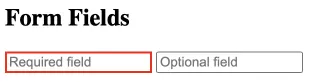
Visit this link to see how it looks in your web browser.
AI Case 2: Attribute Selector with Partial Match
This case study demonstrates the attribute selector with partial match.
Sample AI prompt:
Generate CSS code to style links whose href
attribute starts with "https", changing their color to green.
Sample code output:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Attribute Selector Example 2</title>
<link rel="stylesheet" href="example-2.css" />
</head>
<body>
<h2>Links</h2>
<a href="https://example.com">HTTPS Link</a>
<a href="http://example.com">HTTP Link</a>
</body>
</html>
a[href^="https"] {
color: green;
}
Instructions to see the results:
- Save the code above in
example-2.html
andexample-2.css
. - Open
example-2.html
in your browser to view the external link styled in green, while the internal link remains unstyled.
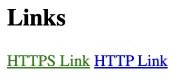
Visit this link to see how it looks in your web browser.
Best Practices for Using CSS Attribute Selectors
CSS attribute selectors provide a flexible way to target elements based on their attributes, allowing you to create efficient, adaptable styles. Here are some best practices to keep in mind:
- Avoid Overuse: Attribute selectors can impact performance in large DOM trees. Use them selectively to avoid slowing down the webpage’s rendering.
- Combine with Other Selectors: Increase specificity by combining attribute selectors with class, ID, or pseudo-classes. This gives you better control over your styling and makes it easier to maintain.
- Use Descriptive Attributes: Choose clear, descriptive attributes for elements in your HTML to make your code more readable and styling more intuitive.
- Optimize for Readability: Keep attribute selectors simple and avoid complex combinations that can make CSS hard to read or debug.
- Test for Compatibility: Some older browsers may not support all attribute selector types. Test your CSS across multiple browsers to ensure compatibility.
By following these best practices, you can create more effective, performant, and maintainable CSS styles with attribute selectors, making your code both robust and adaptable to future changes.