TABLE OF CONTENTS
Django, a high-level Python web framework, has rapidly become a favorite among developers for creating clean, pragmatic, efficient, and easy-to-maintain web applications.
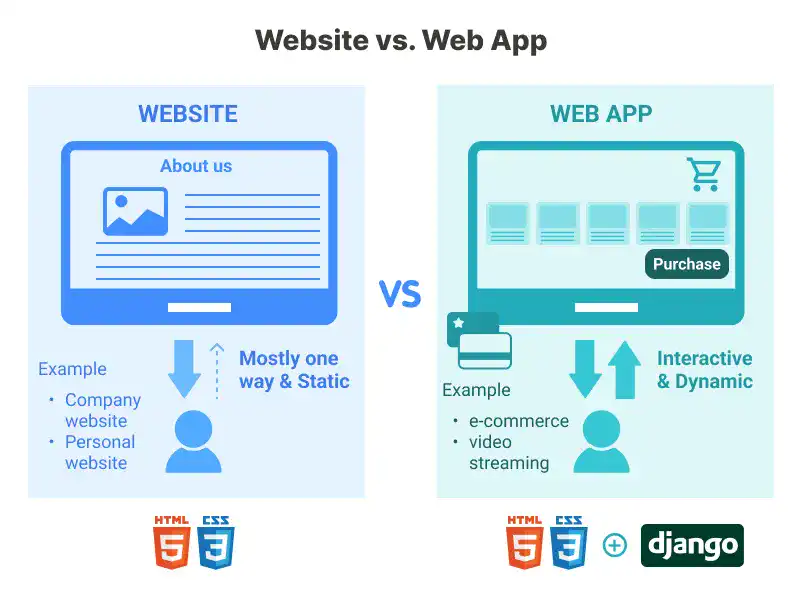
Django’s design encourages rapid development and pragmatic design — two major reasons for its widespread popularity. In this blog, we’ll explore what makes Django stand out and how you can leverage it for your projects.
Understanding Django: The Basics
Django follows the "batteries-included" philosophy and provides almost everything developers might want to do "out of the box." Because of this, it is often referred to as a framework for perfectionists with deadlines.
Django’s architecture is built around the Model-View-Template (MVT) pattern, which is slightly different from the more commonly known Model-View-Controller (MVC) architecture.
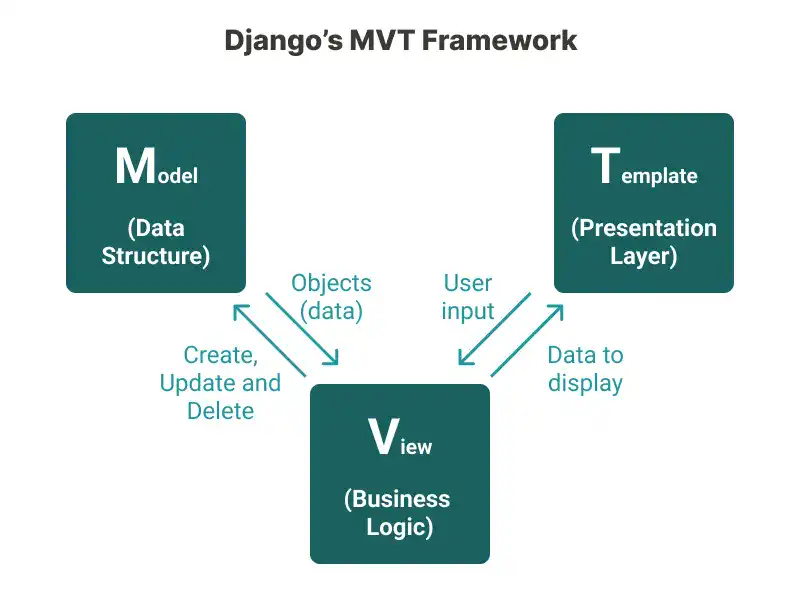
For a closer look at Django's architectural pattern, check out Chapter 1. Django Key Concepts
Quick Start with Django
To begin with Django, you only need a basic understanding of Python.
Setting up a Django project involves a few straightforward commands:
django-admin startproject myproject
cd myproject python
manage.py runserver
This sets up a new Django project and starts a development server. For more detailed guidance on getting your first Django app up and running, refer to Chapter 2. Django Quick Start.
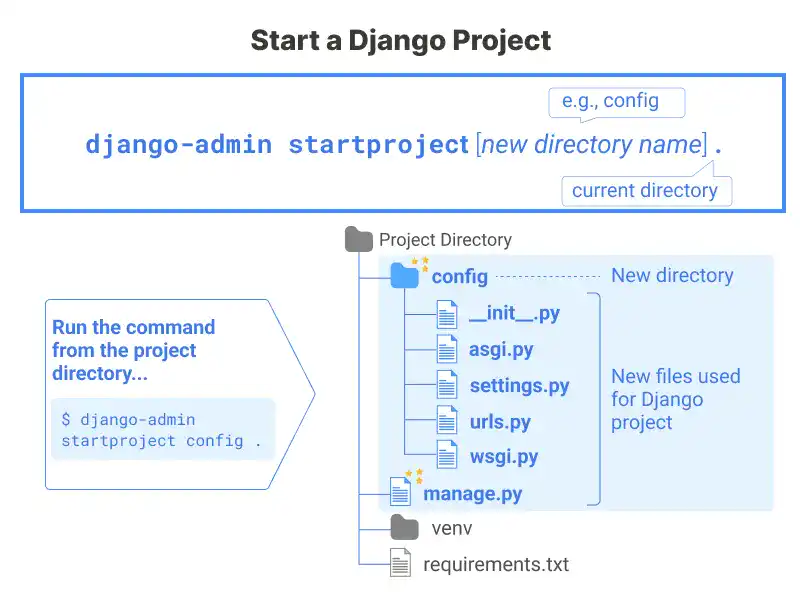
Understanding Django’s Project Structure: Key Files Explained
When you run the django-admin startproject command, it generates several essential files for your Django project. Here’s a brief overview of these key files:
- manage.py: Situated at the root of your project, this script is used to execute various Django commands. It's crucial for running administrative tasks.
- __init__.py: This empty file marks the directory as a Python package, which is a requirement for Python to recognize it as such. Typically, you won’t need to modify this file.
- asgi.py: Defines the ASGI (Asynchronous Server Gateway Interface) application for your project, which is essential for deploying your Django app. Beginners may not need to interact with this file initially.
- settings.py: A vital file where you configure settings for your Django application, such as database configurations and Django-specific options. You will frequently modify this file during development.
- urls.py: Acts as the URL dispatcher for your project, allowing you to define URL patterns linked to responses defined in views.py. Editing and updating this file is common as you develop your app.
- wsgi.py: Implements the WSGI (Web Server Gateway Interface) standard, facilitating communication between your Python application and web servers. This file is important for deployment but may not be immediately relevant for beginners.
Each of these files plays a specific role in the setup and management of your Django project, contributing to its structure and functionality. For an in-depth understanding please refer to our Django Quick Start Guide.
Why Django Is So Popular
Robust and Scalable
Django's ability to handle high traffic and complex data interactions seamlessly is legendary. Sites like Instagram and The Washington Post use Django precisely for its robustness and scalability.
Extensive Documentation and Community
Django boasts one of the most active programming communities and detailed documentation available. This support network makes it an excellent choice for both novice and experienced developers.
Security
Django provides built-in protections against many security threats like SQL injection, cross-site scripting, and forgery, to name a few. Security is such a critical aspect, and you can learn more about how Django handles it in Chapter 5. User Management.
Versatility in Web Applications
Whether you're building a social media site, an e-commerce platform, or a scientific computing platform, Django’s components can be used to build almost any type of web application efficiently. Our Chapter 4. Create CRUD Web Application provides detailed insights into creating a comprehensive web application using Django.
Practical Example: Building a Simple Blog App
Here's a simple Python Django snippet that demonstrates the CRUD operations for a blog application. This example includes the Django models and views required to create, read, update, and delete blog articles.
Models (models.py)
Views (views.py)
Forms (forms.py)
Templates
You'll need to create HTML templates for each view (create_post.html, list_posts.html, detail_post.html, update_post.html, delete_post.html) to enable users to interact with the blog posts. You can learn more about creating templates in the Django Templates for CRUD Views section of our Django learning course.
This snippet shows how Django models streamline interactions with the database for CRUD applications.
Deploying Your Django App
Deployment is the final step of the web development process. Django supports various deployment options, including traditional servers and newer platforms like serverless technologies. For step-by-step instructions on deploying your Django application, see Chapter 6. Deploy Django App.
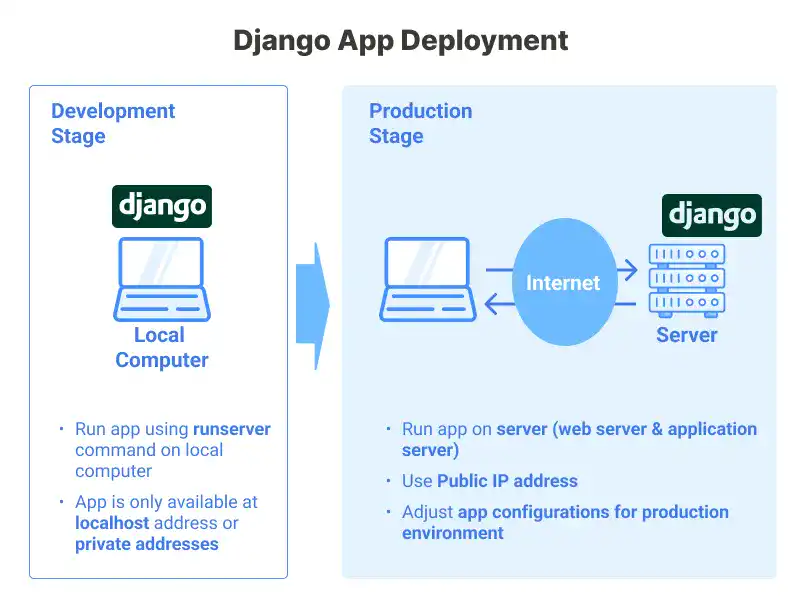
Start Learning Django Today!
Django’s design, built-in features, and robust community make it an outstanding framework for developers looking to build high-quality web applications efficiently. Whether you’re a beginner or an experienced developer, our Django learning course offers a wealth of information to help you master Django.
Ready to dive deeper into Django and build your own web applications? Read our learning course today and start transforming your web development journey with Django. Explore each chapter to gain a deeper understanding and hands-on experience with this powerful framework.
By understanding the fundamental concepts, practical application, and deployment strategies outlined in our learning course, you’ll be well-equipped to take advantage of everything Django has to offer. Don’t miss out on mastering one of today’s most popular web frameworks!