What are the Most Popular Back End Development Languages?
Back-end development is crucial for the functionality of websites and applications, handling everything from server management to database interactions.
This blog post explores the most popular back-end development languages, their unique features, and why they're preferred by developers across the globe. Let's dive into the languages that power the server-side of our applications.
What is Back-End Development?
Back-end development refers to the server-side of software and web development, which focuses on databases, scripting, and website architecture. It is where all the behind-the-scenes magic happens, enabling the front-end to function properly.
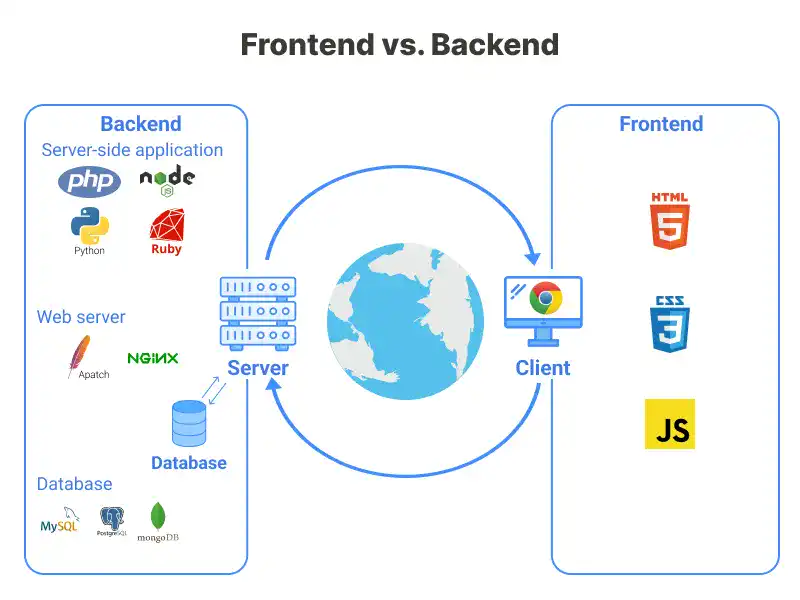
This development layer is crucial for processing and managing data, ensuring everything on the client-side runs smoothly.
Why Back-End Languages Matter
Back-end languages are essential because they help to create the logic and functionality of web applications. They interact with databases, handle user authentication, and ensure that data is served correctly to the user. Mastery of these languages allows developers to build robust, scalable, and secure applications.
Key Back-End Languages
1. Python
Python is a highly versatile and widely used high-level programming language, known for its readability and clean syntax which enables developers to write less code compared to other languages.
Developed by Guido van Rossum and first released in 1991, it supports multiple programming paradigms including procedural, object-oriented, and functional programming.
Python's extensive standard library and third-party packages, accessible through pip, provide tools for a wide range of tasks from web development to data analysis. Its interpreted nature allows for immediate execution of code line by line, which facilitates efficient debugging and iterative testing.
Python's extensive standard library and third-party packages, accessible through pip, provide tools for a wide range of tasks from web development to data analysis. Its interpreted nature allows for immediate execution of code line by line, which facilitates efficient debugging and iterative testing.
Python's application in web development is supported by frameworks like Django and Flask, which help in building scalable web applications. It is also a leading language in data science and machine learning with libraries such as NumPy, pandas, TensorFlow, and PyTorch.
For automation and scripting, Python’s simple syntax is ideal for writing scripts that automate mundane tasks, enhancing workflow efficiency. The language is also prevalently used in academic settings, making it a staple in programming education due to its straightforward syntax and readability.
Key Features:
- Versatile Frameworks: Python works with frameworks such as Django and Flask, which are incredibly powerful for building web applications.
- Wide Range of Libraries: Extensive libraries for everything from machine learning to networking.
- Interpreted Language: Python code is executed line by line, which allows for easy debugging and immediate feedback during development.
- Dynamic Typing: Python is dynamically typed, meaning that the type of a variable is decided at runtime which can increase the flexibility in coding and reduce the setup time significantly.
Benefits:
- Rapid Development: Quick to develop and deploy, making it ideal for startups and prototypes.
- Ease of Learning: Simple to understand, which makes it perfect for beginners.
- Portability: Python code is highly portable and can run on any machine with a Python interpreter, facilitating cross-platform development across Windows, macOS, Linux, and more.
- Community Support: Python boasts a robust and active community that contributed extensively to its ecosystem of libraries and modules, enhancing problem-solving and developer support.
When to Use:
Python is particularly well-suited for data-driven applications, making it a top choice for projects that require extensive data analysis, machine learning, or the handling of large datasets. Its comprehensive selection of data-centric libraries, like pandas for data manipulation and SciPy for scientific computing, simplifies these tasks.
Additionally, Python's straightforward syntax and readability make it ideal for rapid development environments where quick prototyping and iterative testing are crucial. This combination of features ensures that beginners can easily learn and apply Python effectively, especially in projects where time-to-market and agility are key factors.
Code Example:
First, ensure Django is installed in your environment. You can install it using pip if you haven't already:
pip install django
Then, create a new Django project and an app. Here’s how you can set it up quickly:
- Start a new Django project:
django-admin startproject myproject
- Navigate to the project directory:
cd myproject
- Create a new Django app (let's call it webapp):
python manage.py startapp webapp
Now, update the views.py file in your webapp directory to include the following code:
from django.http import HttpResponse def hello_world(request): returnHttpResponse("Hello, from Django!")
Next, you need to hook this view into your project's URL configuration. Open the urls.py file in the myproject directory and modify it as follows:
from django.contrib import admin from django.urls import path from webapp.views importhello_world # make sure to import the view urlpatterns = [ path('admin/', admin.site.urls), path('', hello_world), # Set the hello_world view for the root URL ]
With these changes, your Django application is set up to respond with "Hello, from Django!" when you access the root URL. To see this in action, you can run the development server:
python manage.py runserver
Now, if you visit http://127.0.0.1:8000/ in your web browser, you should see:
Hello, from Django!
This Python code snippet uses Django, a lightweight web application framework. It's setting up a basic route that returns a greeting message.
2. PHP
PHP (Hypertext Preprocessor) is a widely-used, open-source scripting language geared towards web development. It is especially noted for its role in server-side scripting, but can also be used for command line scripting and writing desktop applications. First released in 1995, PHP has evolved significantly over the years and is now a staple in the development of dynamic and interactive websites.
PHP is particularly strong in database interactions and is commonly used in conjunction with SQL databases to create robust content management systems and other web applications that require data manipulation. It is the backbone of popular platforms such as WordPress and Drupal, showcasing its widespread application in content-heavy sites.
For automation and scripting, PHP's simple syntax and powerful integration tools make it ideal for generating dynamic page content and managing server-side tasks efficiently. PHP is also extensively used in academic settings, and its straightforward syntax makes it an accessible entry point for programming education.
Key Features:
- Embedded Capabilities: PHP code can be easily embedded into HTML, making it straightforward to add functionality to web pages without multiple scripts.
- Rich Standard Library: Offers a comprehensive set of built-in functions and classes for tackling a wide array of web development tasks, from file handling to session management.
- Server-Side Scripting: PHP is primarily used for server-side scripting, providing a powerful platform for developing complex web applications.
- Cross-Platform: Runs on various platforms (Windows, Linux, Unix, Mac OS X, etc.) and interfaces with Apache, MySQL, and more, ensuring versatile deployment across multiple systems.
Benefits:
- Cost-Effective: Being open-source, it is free to use and has vast community support, reducing development costs.
- Ease of Learning: PHP syntax is forgiving and easy to learn, which makes it perfect for beginners.
- Efficient Performance: Capable of being as fast as backend languages like Python and Ruby when optimized properly.
- Strong Community Support: Benefits from a vast, active community that contributes to its rich ecosystem of frameworks, libraries, and tools, enhancing problem-solving and developer support.
When to Use:
PHP is particularly effective for developing dynamic websites and web applications that heavily interact with databases. Consider using PHP for projects like e-commerce sites, content management systems, and social media platforms where server-side operations are crucial. The language’s ability to seamlessly integrate with various database systems, coupled with its extensive array of powerful frameworks like Laravel and Symfony, simplifies the development of complex web applications.
Additionally, PHP's long-standing presence and continuous updates make it a reliable choice for long-term projects. For developers looking to quickly prototype and launch web applications, PHP’s wide range of development tools and large ecosystem provide a substantial head start.
Code Example:
Here’s a simple example of creating a new record in a database using PHP with PDO (PHP Data Objects) for a safer, more efficient database interaction:
<?php
$pdo = new PDO('mysql:host=localhost;dbname=test', 'username', 'password');
$statement = $pdo->prepare("INSERT INTO users (username, email) VALUES (:username, :email)");
$statement->execute([
'username' => 'sampleuser',
'email' => 'user@example.com'
]);
echo "User added successfully!";
?>
This PHP snippet demonstrates how to connect to a MySQL database and insert data securely.
3. Ruby
Ruby is a dynamic, high-level programming language valued for its simplicity and productivity, making it a favorite among web developers, particularly for its popular web framework, Ruby on Rails.
Its principle of "least astonishment" means that the language behaves in a way that minimizes confusion for experienced users, enhancing readability and maintainability. Ruby's dynamic typing and automatic memory management allow for concise and flexible code, which can greatly accelerate development timelines.
Ruby is truly object-oriented, with every value being an object, including classes and instances of types that many other languages designate as primitives (like integers and booleans). This uniformity provides a rich, expressive syntax and powerful metaprogramming capabilities.
Ruby's extensive standard library and vibrant ecosystem of gems (libraries) provide developers with tools for a broad spectrum of applications, from simple scripts to complex web applications.
Key Features:
- Dynamic Typing and Duck Typing: Allows for more flexible and less verbose code, which is easier to modify and maintain.
- True Object-Oriented: Every value is an object, which supports highly modular and reusable code.
- Block and Closures: Facilitates functional programming patterns and can simplify complex coding challenges.
- Meta-programming: Ruby's abilities in this area are exceptionally sophisticated, enabling programmers to write more dynamic and abstract code.
Benefits:
- Rapid Development: Reduces development time with its concise syntax and powerful frameworks.
- Flexibility: Adapts to both small scripts and large systems, making it versatile for various types of projects.
- Rich Libraries: A vast array of gems allows rapid integration of new functionalities.
- Strong Community: A supportive and active community contributes to a growing repository of documentation, libraries, and frameworks.
When to Use:
Ruby, and particularly Ruby on Rails, is excellent for startups and rapid application developments where time to market is critical. Its ability to support rapid prototyping and iterate quickly makes it ideal for applications undergoing frequent updates or changes.
For beginners, Ruby's elegant syntax and the supportive community make it an accessible and enjoyable language to learn. Its principles encourage writing clean and maintainable code, which is beneficial for both educational purposes and professional development.
Code Example:
puts "Hello, Ruby!"
Output:
Hello, Ruby!
This simple Ruby script outputs a greeting, demonstrating Ruby’s straightforward syntax and ease of use.
4. Java
Java is a robust, high-level programming language celebrated for its platform independence, making it an ideal choice for back-end development and numerous other applications. Its object-oriented nature supports modular programming, facilitating the breakdown of complex problems into manageable units through objects, enhancing code reusability and maintainability.
Java’s architecture-neutral approach ensures that compiled Java code (in bytecode form) can run on any device equipped with a Java Virtual Machine (JVM), establishing its widespread applicability across different platforms.
Furthermore, Java’s comprehensive standard library equips developers with tools for a variety of programming challenges, from networking to user interface design. Its strong memory management, including automatic garbage collection, helps prevent common programming errors and ensures application stability.
The language’s adherence to strict object-oriented principles and its robust ecosystem of frameworks and tools, like Spring and Hibernate, streamline the development process, enhance functionality, and promote high code quality suitable for enterprise-level applications.
Key Features:
- Platform Independent: Runs on any device that supports Java without the need for recompilation.
- Robust API: Offers a rich set of APIs for activities like networking, XML parsing, and database connection.
- Object-Oriented: Facilitates modular programming and code reuse through its strong support for object-oriented principles.
- Automatic Memory Management: Includes garbage collection, which helps manage memory automatically, reducing the risk of memory leaks and other memory-related issues.
Benefits:
- Scalability: Handles large amounts of data and traffic smoothly.
- Security: Strong security features, making it suitable for applications with sensitive data.
- Stability: Offers a stable platform through its mature ecosystem and thorough pre-deployment code testing.
- Community and Support: Supported by a vast, active community and extensive industry backing, offering numerous resources and tools for developers.
When to Use:
Java is particularly effective for large-scale enterprise applications due to its robust nature, making it a top choice for businesses requiring stable, scalable, and secure software solutions. Its platform independence allows applications to run seamlessly across different operating systems, which is ideal for companies with diverse IT environments.
Additionally, Java’s strong memory management and built-in security features ensure that applications are reliable and safe from various security threats, which is crucial for handling sensitive data.
For beginners, Java's extensive documentation and community support provide a helpful learning path and resources, making it easier to tackle complex projects with confidence. This combination of features makes Java an excellent choice for developing complex business applications that demand high performance and reliability.
Code Example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
Output
Hello Java!
This Java code snippet displays a simple message, showcasing Java’s basic syntax and method structure.
5. C#
C# (pronounced "C-Sharp") is a modern, object-oriented, and type-safe programming language developed by Microsoft. It is part of the .NET framework, which provides a controlled programming environment with robust development tools.
C# is designed to be simple, powerful, safe, and versatile, with features that facilitate both the development of software components suitable for deployment in distributed environments and the development of a wide range of applications—from web to desktop to mobile.
C#’s strong type-checking, array bounds checking, detection of attempts to use uninitialized variables, and automatic garbage collection help to prevent many common software errors. Moreover, its interoperability allows developers to tap into the vast array of libraries developed in other languages like VB.NET and C++.
Key Features:
- Type-Safe: C# enforces strong type-checking which reduces runtime errors and enhances code stability.
- Interoperability: Interacts seamlessly with other languages and technologies on the .NET platform.
- Scalable and Updateable: C# applications are easy to update and scale, adapting to growing business needs.
- LINQ (Language Integrated Query): Integrates directly with the C# language, offering a cohesive query capability for objects, databases, and XML.
Benefits:
- Enhanced Productivity: Features like IntelliSense and powerful debugging tools enhance developer productivity.
- Robust Security: In-built security features protect against various vulnerabilities.
- Rich .NET Library: Offers access to a comprehensive suite of libraries and frameworks.
- Community and Enterprise Support: Strong support from Microsoft and a vast community of developers.
When to Use:
C# is highly recommended for Windows-based applications due to its integration with the .NET framework, making it ideal for desktop applications, games (using Unity), and enterprise-level back-end systems. It’s particularly beneficial for applications that require high levels of security, such as financial or enterprise software.
For beginners, C# provides a clear and strict syntax that can instill good programming practices, backed by extensive documentation and community forums. This makes it suitable for those looking to develop applications in a robust, controlled environment.
Code Example:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, C#!");
}
}
Output:
Hello, C#!
This C# example outputs a simple message, showcasing the basic structure of a C# program and demonstrating how the language uses namespaces
Next Steps to Learn Back-End Development
To further your back-end development skills, consider diving deeper into specialized courses and resources that provide a thorough understanding of programming languages and frameworks. Engaging with D-Libro, our extensive digital library, can significantly enhance your learning journey.
We offer a variety of ebooks and interactive content tailored to both front and back-end development. Particularly, our free Django Introduction course is designed to help beginners and intermediate developers alike understand how to efficiently build robust web applications using this powerful framework. By taking this course, you’ll gain hands-on experience that will prepare you to tackle complex development projects confidently.
Additionally, participating in online communities and contributing to open-source projects can enrich your understanding and provide valuable real-world experience. Start enhancing your skills today by exploring our free Django course and become proficient in back-end development.